In many of my projects I use a custom back button that is only an icon without text. I did some experimenting to find the best way and came up with the below method (I am not saying its the best way or the only way, but it works for me and is stable).
My idea was to implement a Root ViewController that would restyle the standard back-button. Turned out this was not easy at all. After about 1 day of fiddling around with different options I finally came to a working solution.
The trick is to have a transparant background that is BIG enough (bigger then the actual back chevron otherwise this gets resized to smaller size) and then use a custom image for the chevron.
It works, but as you can see on the measurements below, it has a small disadvantage: there is an extra 14 points of gap on the left. If you want matching buttons on the right, you will need to compensate this by 14 points (and yes its points so on retina this is 28 pixels...)
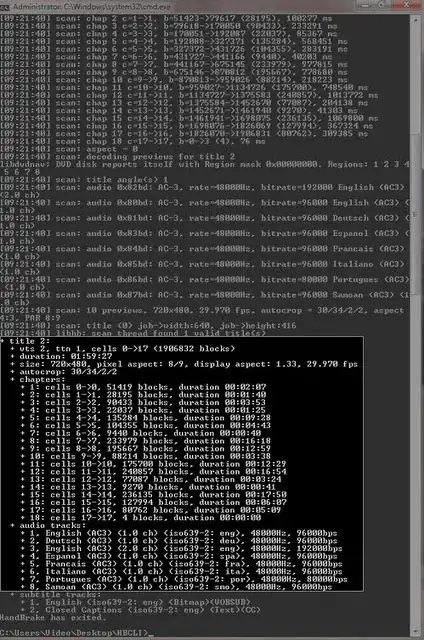
This is my code:
//this vc implements a custom back button, you can make this a root controller
//from which you inherit all your view controllers. But for simplicity reasons for this explanation
//I skipped this
class VCRoot: UIViewController {
override func viewDidLoad() {
//call supers
super.viewDidLoad()
//create custom back item
let backItem = UIBarButtonItem()
//as image set the back chevron icon its a 22x22 points (so 44x44 in retina)
backItem.image=PaintCode.imageOfBarBtnBack
let b = PaintCode.imageOfBarBtnBackgroundEmpty
//as background I use a 1 = 33 points (so 2x66 retina) fully transparant image
backItem.setBackButtonBackgroundImage(b, forState: UIControlState.Normal, barMetrics: UIBarMetrics.Default)
//set the newly created barbuttonitme as the back button
navigationItem.backBarButtonItem=backItem
}
}
//this vc puts a "forwards" button in the navbar on the right with a matching arrow
//The image of this matching forward arrow is the correct size (width!) so that its the same
//distance from the edge of the screen as the back button
class VC2: UIViewController {
//outlet to the barbutton item from IB
@IBOutlet weak var barbtn: UIBarButtonItem!
override func viewDidLoad() {
//call supers
super.viewDidLoad()
//the forward chevron in the image is shifted 14 points (so 28 retina) to the left
//so it has same distance from edge => its (22+14) x 22 = 36 x 22 points (72 x 44 retina)
barbtn.image=PaintCode.imageOfBarBtnForward
}
}
You can also refer to my blog here :
http://www.hixfield.net/blog/2015/05/adding-a-custom-back-button-the-standard-way/