Answers above always mentioned the same problem like:
there is the possibility the download could complete before you attach the onload event handler
or
but if setting the src and the image loads before your handler is attached, it won't fire.
or bug with IE7.
First, let's ignore IE7.
Second, I don't think problem mentioned exist, for example:
function loadImg(url) {
let img = new Image()
img.src = url
let date1 = Date.now()
console.log(img.complete)
while (Date.now() - date1 < 5000) {
}
img.onload = function() {
console.log('success')
}
console.log('sync first')
}
loadImg('https://cdn.sstatic.net/Sites/stackoverflow/img/sprites.svg')
Normally, you will get:
false
sync first
success
Well, if you executed on another site which will use cache more than one time. You will get the result like image below:
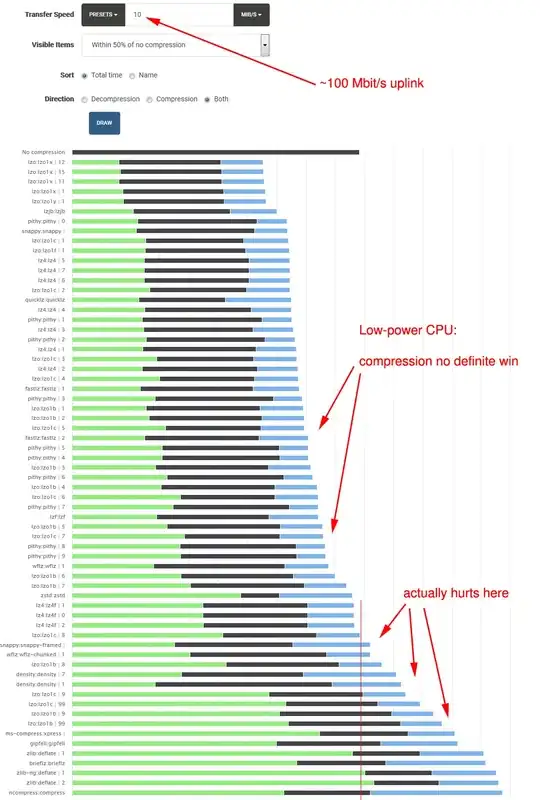
The answer is clear now.
Well, as long as you set the onload
synchronously. You will not miss the onload
event.
Why would I say synchronously
. For another example,
function loadImg(url) {
let img = new Image()
img.src = url
let date1 = Date.now()
console.log(img.complete)
while (Date.now() - date1 < 5000) {
}
setTimeout(function() {
img.onload = function() {
console.log('success')
}
})
console.log('sync first')
}
loadImg('https://cdn.sstatic.net/Sites/stackoverflow/img/sprites.svg')
The result on another site:
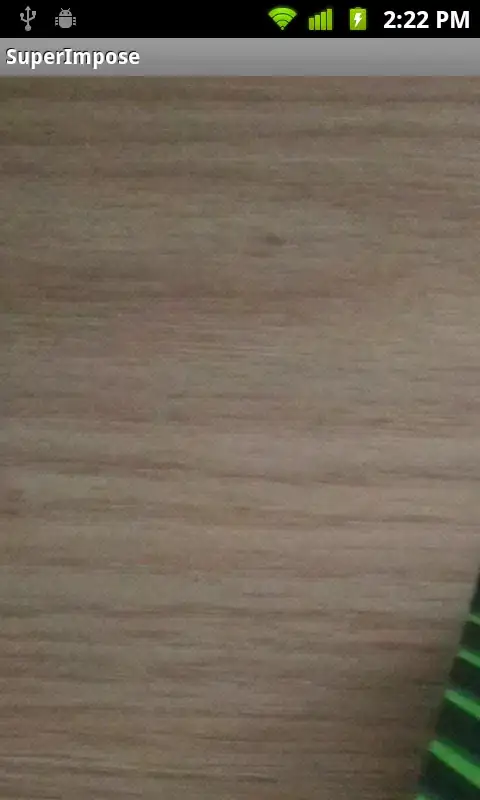
The second time with cache, load
event won't be triggered. And the reason is setTimeout
is asynchronously.