I wonder why such a simple and trivial things as handling date/time objects is that complicated
The information technology industry as a whole went decades without tackling the surprisingly tricky problem of date-time handling.
Java originally inherited some code provided by Taligent/IBM. These became the java.util.Date
,Calendar
, GregorianCalendar
, and related classes. They are terrible, bloody awful, flawed in design, and not object-oriented.
The Joda-Time project led by Stephen Colebourne was the first attempt I know of to tackle the date-time problem in a robust and competent manner. Later, Colebourne et al. took the lessons learned there to lead JSR 310, the java.time implementation, and related projects, the back-port in ThreeTen-Backport, and further additional functionality in ThreeTen-Extra.
So now Java has the industry-leading framework for date-time work in the java.time classes.
object which holds the parameters for Day, Month, Year, Hour, Minute, Second... and the possibility to manipulate theses values.
For a date-only, use LocalDate
. For a date with time-of-day but lacking the context of a known offset-from-UTC or time zone, use LocalDateTime
(not a moment). For a moment, a specific point on the timeline, use Instant
(always in UTC), OffsetDateTime
(a date with time-of-day in the context of a certain number of hours-minutes-seconds ahead/behind the prime meridian), or ZonedDateTime
(a date and time with a time zone assigned).
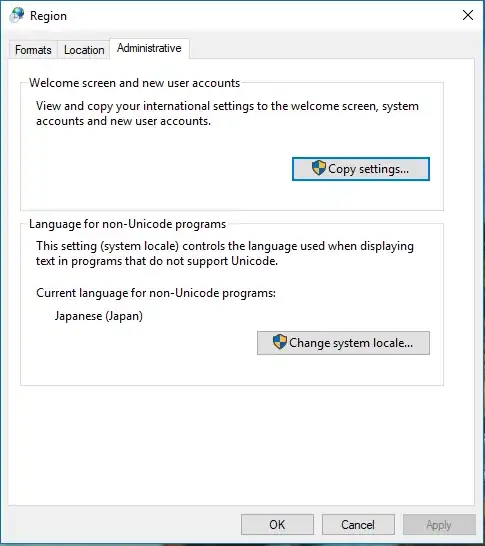
Question 1: How to create a date object from minutes?
Not sure what you mean.
To represent a span-of-time unattached to the timeline, use Duration
.
Duration d = Duration.ofMinutes( 10 ) ;
Instant now = Instant.now() ;
Instant tenMinutesFromNow = now.plus( d ) ;
If you have a count of minutes into the day, use LocalTime.MIN
(00:00:00) with a Duration
.
Duration d = Duration.ofMinutes( 10 ) ;
LocalTime lt = LocalTime.MIN.plus( d ) )
Question 2: How to create a date object from int's??
I am guessing you mean you want specify the year, month, day, and so on as a series of numbers. For that, use the LocalDateTime
factory methad.
LocalDateTime ldt = LocalDateTime.of( 2020 , 1 , 23 , 15 , 30 , 0 , 0 ) ; // year, month, day, hour, minute, second, nanos fraction of second.
Or build up from a LocalDate
and LocalTime
.
LocalDate ld = LocalDate.of( 2020 , 1 , 23 ) ;
LocalTime lt = LocalTime.of( 15 , 30 ) ;
LocalDateTime ldt = LocalDateTime.of( ld , lt ) ;
As mentioned above, a LocalDateTime
cannot represent a moment. To determine a moment, specify a time zone. For example, in our code above, do you mean 3:30 PM in Tokyo, Toulouse, or Toledo — thee different moments, several hours apart.
ZoneId z = ZoneId.of( "Asia/Tokyo" ) ;
ZonedDateTime zdt = ZonedDateTime.of( ld , lt , z ) ;
Adjust into UTC by extracting a Instant
.
Instant instant = zdt.toInstant() ;
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes.
Where to obtain the java.time classes?
The ThreeTen-Extra project extends java.time with additional classes. This project is a proving ground for possible future additions to java.time. You may find some useful classes here such as Interval
, YearWeek
, YearQuarter
, and more.