I can see the sense of using a game API as suggested by @Hovercraft, but feel that many simple games can do without it.
I agree with the first 2 points of @Guillaume, but would tend to go in a different direction after that.
Let us assume the game:
- Is a fixed size (i.e. non-resizable).
- Has no components appearing over it.
In that case, I would tend to do the rendering in a BufferedImage
that is displayed in a label.
Answers to specific questions
Should I use Swing JFrame and than add it a Graphics object?
That would not compile. So ..no.
Should I make a panel first and add the graphics on to it instead?
That is basically what Guillaume is suggesting, though I prefer using an image as the canvas on which to paint.
Or maybe should I use a canvas instead of a JPanel?
If by 'canvas' you mean java.awt.Canvas
then no.
If you mean a java.awt.image.BufferedImage
then yes.
Some advise to use the paint()
method while others demonstrates code while using the paintComponent()
...
This is a common confusion since there is so much old and bad code out there in the World Wild Web.
The only components that overriding paint(Graphics)
would work with are components of the AWT & Swing top-level containers such as JFrame
, JApplet
or JWindow
.
- AWT is last millennium's GUI component toolkit. We should use Swing this millennium.
- It is unusual for an entire GUI to be custom painted. Instead it might be added as the major component amongst others that report player health, lives remaining, score etc. For that (and other) reasons, it is better to render to a 'non top-level container' which would leave us looking at doing custom painting in a
JComponent
or JPanel
.
For custom painting in an extended panel or component, override paintComponent(Graphics)
. That is the correct way to do custom painting for those components.
Other tips
Each object of the game (e.g. Ship
, Enemy
, Missile
) should know how to draw itself. Keep a reference to each of the game elements in the painting routine, and simply call gameElement.paint(Graphics)
(or Graphics2D
) on the instance of graphics it is painting.
If the game elements are drawn from Java-2D based Shape
instances, or if a Shape
can be defined from the existing sprite images, collision detection becomes simple. For details see:
- This answer to Get mouse detection with a dynamic shape
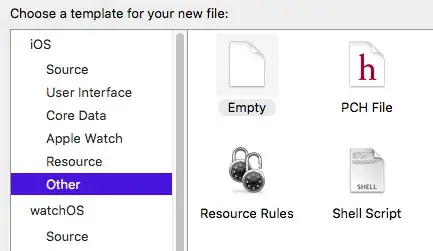
- This answer to Collision detection with complex shapes
