Maybe you want to set the xticks
:
import pylab as pl
data = genfromtxt('myfile.dat')
pl.axis('auto')
xs = pl.arange(data.shape[0])
pl.plot(xs, data[:,0])
pl.xticks(xs, data[:,1])
Working sample:
Another option would be to work with datetimes. If you work with dates, you can use those as input to the plot command.
Working sample:
import random
import pylab as plt
import datetime
from matplotlib.dates import DateFormatter, DayLocator
fig, ax = plt.subplots(2,1, figsize=(6,8))
# Sample 1: use xticks
days = [29,30,31,1,2,3,4,5]
values = [random.random() for x in days]
xs = range(len(days))
plt.axes(ax[0])
plt.plot(xs, values)
plt.xticks(xs, days)
# Sample 2: Work with dates
date_strings = ["2013-01-30",
"2013-01-31",
"2013-02-01",
"2013-02-02",
"2013-02-03"]
dates = [datetime.datetime.strptime(x, "%Y-%m-%d") for x in date_strings]
values = [random.random() for x in dates]
plt.axes(ax[1])
plt.plot(dates,values)
ax[1].xaxis.set_major_formatter(DateFormatter("%b %d"))
ax[1].xaxis.set_major_locator(DayLocator())
plt.show()
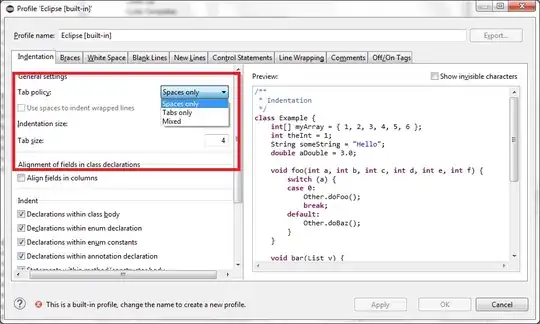