Given what is k-arrangement (http://en.wikibooks.org/wiki/Probability/Combinatorics), you are looking for the k-arrangement where k varies from 1 to D, where D is the size of the data collections.
This means to compute - my first post I can't post image so look at equation located at :
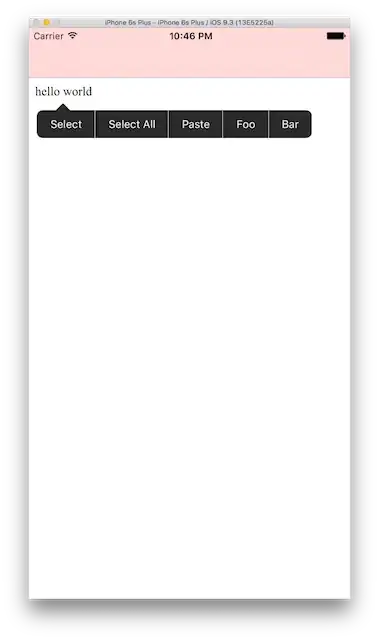
In order to do it, you can make k varies, and the for each k may n varies (i.e. deal only with a sub array or data to enumerate the k-permutations). These k-permutations can be found by walking the array to the right and to the left using recursion.
Here is a quick bootstrap that proves to enumerate whart is required :
public class EnumUrl {
private Set<String> enumeration = null;
private List<String> data = null;
private final String baseUrl = "http://localhost:8080/service/USERID=101556000/";
public EnumUrl(List<String> d) {
data = d;
enumeration = new HashSet<String>(); // choose HashSet : handle duplicates in the enumeration process
}
public Set<String> getEnumeration() {
return enumeration;
}
public static void main(String[] args) {
List<String> data = new ArrayList<String>();
data.add("A");
data.add("B");
data.add("C");
EnumUrl enumerator = new EnumUrl(data);
for (int k = 0; k < data.size(); k++) {
// start from any letter in the set
for (int i = 0; i < data.size(); i++) {
// enumerate possible url combining what's on the right of indice i
enumerator.enumeratePossibleUrlsToRight(data.get(i), i);
// enumerate possible url combining what's on the left of indice i
enumerator.enumeratePossibleUrlsToLeft(data.get(i), i);
}
// make a circular permutation of -1 before the new iteration over the newly combined data
enumerator.circularPermutationOfData();
}
// display to syso
displayUrlEnumeration(enumerator);
}
private void circularPermutationOfData() {
String datum = data.get(0);
for (int i = 1; i < data.size(); i++) {
data.set(i - 1, data.get(i));
}
data.set(data.size() - 1, datum);
}
private static void displayUrlEnumeration(EnumUrl enumerator) {
for (String url : enumerator.getEnumeration()) {
System.out.println(url);
}
}
private void enumeratePossibleUrlsToRight(String prefix, int startAt) {
enumeration.add(baseUrl + prefix);
if (startAt < data.size() - 1) {
startAt++;
for (int i = startAt; i < data.size(); i++) {
int x = i;
enumeratePossibleUrlsToRight(prefix + "," + data.get(x), x);
}
}
}
private void enumeratePossibleUrlsToLeft(String prefix, int startAt) {
enumeration.add(baseUrl + prefix);
if (startAt > 0) {
startAt--;
for (int i = startAt; i >= 0; i--) {
int x = i;
enumeratePossibleUrlsToLeft(prefix + "," + data.get(x), x);
}
}
}
}
The program outputs for {A,B,C} :
http://localhost:8080/service/USERID=101556000/B,C
http://localhost:8080/service/USERID=101556000/B,A,C
http://localhost:8080/service/USERID=101556000/B,C,A
http://localhost:8080/service/USERID=101556000/B,A
http://localhost:8080/service/USERID=101556000/C
http://localhost:8080/service/USERID=101556000/B
http://localhost:8080/service/USERID=101556000/C,B,A
http://localhost:8080/service/USERID=101556000/A,C,B
http://localhost:8080/service/USERID=101556000/A,C
http://localhost:8080/service/USERID=101556000/A,B
http://localhost:8080/service/USERID=101556000/A,B,C
http://localhost:8080/service/USERID=101556000/A
http://localhost:8080/service/USERID=101556000/C,B
http://localhost:8080/service/USERID=101556000/C,A
http://localhost:8080/service/USERID=101556000/C,A,B
And for {A,B,C,D} :
http://localhost:8080/service/USERID=101556000/B,A,D,C
http://localhost:8080/service/USERID=101556000/C,D
http://localhost:8080/service/USERID=101556000/A,D,C,B
http://localhost:8080/service/USERID=101556000/A,C,D
http://localhost:8080/service/USERID=101556000/D
http://localhost:8080/service/USERID=101556000/C
http://localhost:8080/service/USERID=101556000/A,C,B
http://localhost:8080/service/USERID=101556000/B
http://localhost:8080/service/USERID=101556000/A,B,C,D
http://localhost:8080/service/USERID=101556000/A,B,C
http://localhost:8080/service/USERID=101556000/D,C,B,A
http://localhost:8080/service/USERID=101556000/C,B,A,D
http://localhost:8080/service/USERID=101556000/A,B,D
http://localhost:8080/service/USERID=101556000/D,B
http://localhost:8080/service/USERID=101556000/D,C
http://localhost:8080/service/USERID=101556000/A
http://localhost:8080/service/USERID=101556000/D,C,A
http://localhost:8080/service/USERID=101556000/D,C,B
http://localhost:8080/service/USERID=101556000/C,D,A
http://localhost:8080/service/USERID=101556000/C,D,B
http://localhost:8080/service/USERID=101556000/D,A
http://localhost:8080/service/USERID=101556000/A,D,C
http://localhost:8080/service/USERID=101556000/A,D,B
http://localhost:8080/service/USERID=101556000/C,B,D
http://localhost:8080/service/USERID=101556000/B,A,D
http://localhost:8080/service/USERID=101556000/B,C
http://localhost:8080/service/USERID=101556000/B,A,C
http://localhost:8080/service/USERID=101556000/B,C,A
http://localhost:8080/service/USERID=101556000/B,A
http://localhost:8080/service/USERID=101556000/B,C,D
http://localhost:8080/service/USERID=101556000/C,B,A
http://localhost:8080/service/USERID=101556000/A,D
http://localhost:8080/service/USERID=101556000/D,A,B
http://localhost:8080/service/USERID=101556000/A,C
http://localhost:8080/service/USERID=101556000/D,A,C
http://localhost:8080/service/USERID=101556000/B,C,D,A
http://localhost:8080/service/USERID=101556000/A,B
http://localhost:8080/service/USERID=101556000/B,D
http://localhost:8080/service/USERID=101556000/C,D,A,B
http://localhost:8080/service/USERID=101556000/D,A,B,C
http://localhost:8080/service/USERID=101556000/D,B,A
http://localhost:8080/service/USERID=101556000/D,B,C
http://localhost:8080/service/USERID=101556000/B,D,A
http://localhost:8080/service/USERID=101556000/C,B
http://localhost:8080/service/USERID=101556000/C,A,D
http://localhost:8080/service/USERID=101556000/C,A
http://localhost:8080/service/USERID=101556000/B,D,C
http://localhost:8080/service/USERID=101556000/C,A,B
Which is not the exhaustive enumeration. Basically we should have:
(my first post I can't post image to look at equation located in my reply, I don't have the reputation to post 2 links ... #omg)
That makes 64 combinaisons, distributed as follows:
- 4 combinaisons of 1 element (k=1)
- 12 combinaisons of 12 element (k=2)
- 24 combinaisons of 24 element (k=3)
- 24 combinaisons of 24 element (k=4)
You can see that my program is OK for k=1, k=2, and k=3. But there are not 24 combinaisons for k=4. In order to complete the program, you will need to iterate also on other type of shuffling the data than circular permutation. Actually when k=4, circular permutation does not generate for instance ADBC as input data (hence DBCA cannot be generated by my implementation for instance). In this case, you will want to enumerate all possible data input array with n elements in all possible order. This is a special case of k-permutation, where k=n, and therefore leads to finding the n!
permutation. We can achieve this by calling the EnumUrl method for each of the n!
possible permutation.
For this, you should update EnumUrl enumerator = new EnumUrl(data);
accordingly, but I guess I am letting some work for you to make :-)
HTH