This seems to work. Note to use the same instance of Random
! The 1st image limits the B of the HSB to .5f, while 2nd image shows the effect of using Color.darker()
instead.
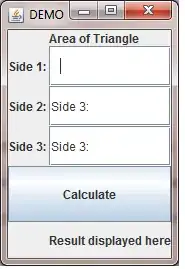
import java.awt.*;
import java.awt.image.BufferedImage;
import javax.swing.*;
import java.util.Random;
import java.util.logging.*;
import javax.imageio.ImageIO;
import java.io.*;
class DarkHSB {
float darker = .5f;
Random r = new Random();
public final Color generateRandomColor(boolean useHsbApi) {
float brightness = (useHsbApi ? r.nextFloat() * darker : r.nextFloat());
// Random objects created sequentially will have the same seed!
Color c = Color.getHSBColor(
r.nextFloat(),
r.nextFloat(),
brightness);
if (!useHsbApi) c = c.darker();
return c;
}
public void paint(Graphics g, int x, int y, int w, int h, boolean useApi) {
g.setColor(generateRandomColor(useApi));
g.fillRect(x,y,w,h);
}
public static void main(String[] args) {
Runnable r = new Runnable() {
@Override
public void run() {
final JPanel gui = new JPanel(new GridLayout(0,1));
final DarkHSB dhsb = new DarkHSB();
int w = 300;
int h = 100;
BufferedImage hsb = new BufferedImage(w,h,BufferedImage.TYPE_INT_RGB);
Graphics g = hsb.getGraphics();
int sz = 5;
for (int xx=0; xx<w; xx+=sz) {
for (int yy=0; yy<h; yy+=sz) {
dhsb.paint(g,xx,yy,sz,sz,true);
}
}
g.dispose();
BufferedImage darker = new BufferedImage(w,h,BufferedImage.TYPE_INT_RGB);
g = darker.getGraphics();
for (int xx=0; xx<w; xx+=sz) {
for (int yy=0; yy<h; yy+=sz) {
dhsb.paint(g,xx,yy,sz,sz,false);
}
}
g.dispose();
gui.add(new JLabel(new ImageIcon(hsb)));
gui.add(new JLabel(new ImageIcon(darker)));
JOptionPane.showMessageDialog(null, gui);
File userHome = new File(System.getProperty("user.home"));
File img = new File(userHome,"image-hsb.png");
dhsb.saveImage(hsb,img);
img = new File(userHome,"image-darker.png");
dhsb.saveImage(darker,img);
}
};
// Swing GUIs should be created and updated on the EDT
// http://docs.oracle.com/javase/tutorial/uiswing/concurrency/initial.html
SwingUtilities.invokeLater(r);
}
public void saveImage(BufferedImage bi, File file) {
try {
ImageIO.write(bi, "png", file);
} catch (IOException ex) {
Logger.getLogger(DarkHSB.class.getName()).log(Level.SEVERE, null, ex);
}
}
}