Here is a sample. I hope it will get you keep going.
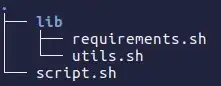
<asp:ListView runat="server" ID="ListView1" ItemPlaceholderID="Panel1"
OnItemDataBound="ListView_ItemDataBound">
<LayoutTemplate>
<asp:Panel runat="server" ID="Panel1"></asp:Panel>
</LayoutTemplate>
<ItemTemplate>
<%#Eval("Name") %>
<asp:GridView runat="server" ID="GridView1" AutoGenerateColumns="False">
<Columns>
<asp:BoundField DataField="LastName" HeaderText="LastName" />
<asp:BoundField DataField="FirstName" HeaderText="FirstName" />
</Columns>
</asp:GridView>
</ItemTemplate>
</asp:ListView>
public class House
{
public int HouseId { get; set; }
public string Name { get; set; }
public List<User> Users { get; set; }
}
public class User
{
public int UserId { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
}
public List<House> Houses
{
get
{
return new List<House>
{
new House
{
HouseId = 1,
Name = "House One",
Users = new List<User>()
{
new User {UserId = 1, FirstName = "John", LastName = "Newton"},
new User {UserId = 2, FirstName = "Marry", LastName = "Newton"},
new User {UserId = 3, FirstName = "Joe", LastName = "Newton"}
}
},
new House
{
HouseId = 1,
Name = "House Two",
Users = new List<User>()
{
new User {UserId = 6, FirstName = "Newton", LastName = "Doe"},
new User {UserId = 7, FirstName = "Jack", LastName = "Doe"},
new User {UserId = 8, FirstName = "Lewis", LastName = "Doe"}
}
}
};
}
}
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
ListView1.DataSource = Houses;
ListView1.DataBind();
}
}
protected void ListView_ItemDataBound(object sender, ListViewItemEventArgs e)
{
var house = e.Item.DataItem as House;
var gridView = e.Item.FindControl("GridView1") as GridView;
gridView.DataSource = house.Users;
gridView.DataBind();
}