For fetching the array items and show it into a listview:
ArrayList titles = new ArrayList();
ArrayList descs= new ArrayList();
ArrayList links= new ArrayList();
for (int i = 0; i < titlesObj.length(); i++) {
String title = titlesObj.getString(i);
titles.add(title);
System.out.println("Titles: " + title);
}
for (int i = 0; i < descsObj.length(); i++) {
String desc = descsObj.getString(i);
descs.add(title);
System.out.println("Desc: " + desc);
}
for (int i = 0; i < linksObj.length(); i++) {
String link = linksObj.getString(i);
links.add(title);
System.out.println("Link: " + link);
}
Next you make this arraylist a source to a listview like this:
// Get a handle to the list views
//get your instance of the listview for titles
ListView lvTitle = (ListView) findViewById(R.id.ListView01);
lv.setAdapter(new ArrayAdapter<string>((Your activity class).this,
android.R.layout.simple_list_item_1, titles ));
//get your instance of the listview for descriptions
ListView lvDesc = (ListView) findViewById(R.id.ListView02);
lv.setAdapter(new ArrayAdapter<string>((Your activity class).this,
android.R.layout.simple_list_item_1, descs));
//get your instance of the listview for links
ListView lvLinks = (ListView) findViewById(R.id.ListView03);
lv.setAdapter(new ArrayAdapter<string>((Your activity class).this,
android.R.layout.simple_list_item_1, links));
Edit
Whatever I said that should already solve your question. But, I can see that you are using the same activity to communicate to a remote server to get data. I suggest it would be best if you make a separate class for this which would return the json text data
. Then you can call this class from your activity to get data and set the list views in your activity. It would avoid unnecessary lags and force closes in your app.
Update
You need to implement a custom adapter for that. You need to define a single listitem.xml
with Layouts according to your requirement. Then inflate it to the list view.
Follow this tutorial.
Sample Row:
list_row.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:background="@drawable/list_selector"
android:orientation="horizontal"
android:padding="5dip" >
<!-- ListRow Left sied Thumbnail product image -->
<LinearLayout android:id="@+id/thumbnail"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="3dip"
android:layout_alignParentLeft="true"
android:background="@drawable/image_bg"
android:layout_marginRight="5dip">
<ImageView
android:id="@+id/list_image"
android:layout_width="50dip"
android:layout_height="50dip"
android:src="@drawable/someImage"/>
</LinearLayout>
<!-- Your title-->
<TextView
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignTop="@+id/thumbnail"
android:layout_toRightOf="@+id/thumbnail"
android:text="Big Title"
android:textColor="#040404"
android:typeface="sans"
android:textSize="15dip"
android:textStyle="bold"/>
<!-- Your subtitle -->
<TextView
android:id="@+id/subtitle"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_below="@id/title"
android:textColor="#343434"
android:textSize="10dip"
android:layout_marginTop="1dip"
android:layout_toRightOf="@+id/thumbnail"
android:text="Smaller sub title" />
<!-- Rightend info -->
<TextView
android:id="@+id/duration"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentRight="true"
android:layout_alignTop="@id/title"
android:gravity="right"
android:text="info"
android:layout_marginRight="5dip"
android:textSize="10dip"
android:textColor="#10bcc9"
android:textStyle="bold"/>
<!-- Rightend Arrow -->
<ImageView android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/arrow"
android:layout_alignParentRight="true"
android:layout_centerVertical="true"/>
</RelativeLayout>
which would make your list row look like:
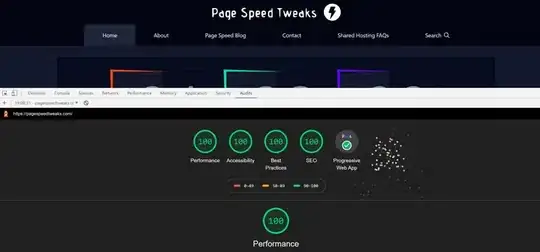