If you want to have table with table schema, table name, column name and description you can use following query:
select TableName = tbl.table_schema + '.' + tbl.table_name,
sc.name [Column],
sep.value [Description]
from sys.tables st
inner join information_schema.tables tbl on st.object_id=object_id(tbl.table_schema + '.' + tbl.table_name)
inner join sys.columns sc on st.object_id = sc.object_id
left join sys.extended_properties sep on st.object_id = sep.major_id
and sc.column_id = sep.minor_id
and sep.name = 'MS_Description'
This will generate follwing table:
Table name |
Column name |
Description |
dbo.VersionInfo |
AppliedOn |
Description |
I wanted to generate the md file in my CI/CD pipeline and for this purpose I created a PowerShell module. It has Out-Md switch which generates MD file with all descriptions.
Get-ColumnsDescription -Verbose -ServerInstance ".\SQL2019" -Database PTMeetings -OutMD
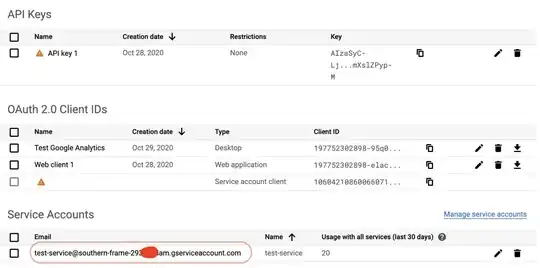
To cover topic completely:
If you would like to add the description with the query you can use
EXEC sys.sp_addextendedproperty
@name=N'MS_Description' --name of property
,@value=N'Age should be between 12 and 100 Descp' --descption text
,@level0type=N'SCHEMA'
,@level0name=N'jl' --Schema name
,@level1type=N'TABLE'
,@level1name=N'JournalItemNotes' --Table Name
,@level2type=N'COLUMN'
,@level2name=N'NotesType' -- Column Name
And if You are interested in the idempotent function I propose to create procedure:
IF OBJECT_ID ('dbo.usp_addorupdatedescription', 'P') IS NOT NULL
DROP PROCEDURE dbo.usp_addorupdatedescription;
GO
CREATE PROCEDURE usp_addorupdatedescription
@table nvarchar(128), -- table name
@column nvarchar(128), -- column name, NULL if description for table
@descr sql_variant -- description text
AS
BEGIN
SET NOCOUNT ON;
DECLARE @c nvarchar(128) = NULL;
IF @column IS NOT NULL
SET @c = N'COLUMN';
BEGIN TRY
EXECUTE sp_updateextendedproperty N'MS_Description', @descr, N'SCHEMA', N'dbo', N'TABLE', @table, @c, @column;
END TRY
BEGIN CATCH
EXECUTE sp_addextendedproperty N'MS_Description', @descr, N'SCHEMA', N'dbo', N'TABLE', @table, @c, @column;
END CATCH;
END
GO