This is technically possible if you run with the debugger. You didn't tell the whole story though. The debugger will display a dialog first when the exception is thrown. It looks similar to this (mine is VS2012 on Windows 8):
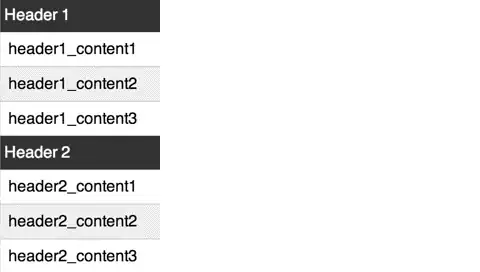
If you click the Break button then the debugger will break at the throw statement, giving you a chance to inspect state. When you click the Continue button then the exception is ignored and the program will continue as though nothing happened. This also happens when you break and then resume running.
This is a feature, not a bug, it allows you to rescue a debug session on which you already spent a lot of time. It is of course not often that useful to continue since your program is likely to be in a bad state but the alternative isn't great either. You almost always want to favor clicking Break and use the debugger to correct state so that you can meaningfully continue debugging.
Of course this will never happen without a debugger, your program will instantly terminate.
The only possible way that a stack unwind is ever going to happen is by writing a catch clause that catches the exception. Regardless of whether you are debugging or not.