Find a LatLng at a distance of radius units from center LatLng on Map
now convert both these LatLngs to screenCoordinates
Use the formula used to construct a cirle x = Rsin(theta) , y = Rcos(theta)
you divide the circle into N segments and then draw polylines(drawn on map) on the circumference of the circle converting the screen coordinates to LatLngs
more the number of N more it looks like a circle , I have used N = 120 according the zoom level ,I am using 13.
private void addDottedCircle(double radius) {//radius is in kms
clearDottedCircle();
LatLng center,start,end;
Point screenPosCenter,screenPosStart,screenPosEnd;
Projection p = mMap.getProjection();
center = searchCenterMarker.getPosition();
start = new LatLng(center.latitude + radius/110.54,center.longitude);
// radius/110.54 gives the latitudinal delta we should increase so that we have a latitude at radius distance
// 1 degree latitude is approximately 110.54 kms , so the above equation gives you a rough estimate of latitude at a distance of radius distance
screenPosCenter = p.toScreenLocation(center);
screenPosStart = p.toScreenLocation(start);
double R = screenPosCenter.y - screenPosStart.y;
int N = 120;//N is the number of parts we are dividing the circle
double T = 2*Math.PI/N;
double theta = T;
screenPosEnd = new Point();
screenPosEnd.x = (int)(screenPosCenter.x-R*Math.sin(theta));
screenPosEnd.y = (int) (screenPosCenter.y-R*Math.cos(theta));
end = p.fromScreenLocation(screenPosEnd);
for(int i =0;i<N;i++){
theta+=T;
if(i%2 == 0){
//dottedCircle is a hashmap to keep reference to all the polylines added to map
dottedCircle.add(mMap.addPolyline(new PolylineOptions().add(start,end).width(5).color(Color.BLACK)));
screenPosStart.x = (int) (screenPosCenter.x-R*Math.sin(theta));
screenPosStart.y = (int) (screenPosCenter.y-R*Math.cos(theta));
start = p.fromScreenLocation(screenPosStart);
}
else{
screenPosEnd.x = (int)(screenPosCenter.x-R*Math.sin(theta));
screenPosEnd.y = (int) (screenPosCenter.y-R*Math.cos(theta));
end = p.fromScreenLocation(screenPosEnd);
}
}
}
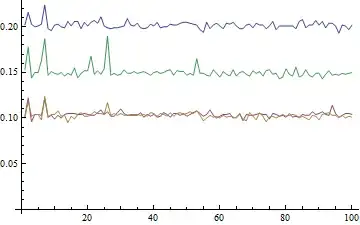