Ok, this is my take on a ChessBoard:
<Window x:Class="MiscSamples.ChessBoard"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:MiscSamples"
Title="ChessBoard" Height="300" Width="300">
<Window.Resources>
<DataTemplate DataType="{x:Type local:ChessPiece}">
<Image Source="{Binding ImageSource}"/>
</DataTemplate>
</Window.Resources>
<Grid>
<UniformGrid Rows="8" Columns="8" Opacity=".5">
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
<Rectangle Fill="Black"/>
<Rectangle Fill="White"/>
</UniformGrid>
<ItemsControl ItemsSource="{Binding}">
<ItemsControl.ItemsPanel>
<ItemsPanelTemplate>
<Grid IsItemsHost="True">
<Grid.RowDefinitions>
<RowDefinition/>
<RowDefinition/>
<RowDefinition/>
<RowDefinition/>
<RowDefinition/>
<RowDefinition/>
<RowDefinition/>
<RowDefinition/>
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition/>
<ColumnDefinition/>
<ColumnDefinition/>
<ColumnDefinition/>
<ColumnDefinition/>
<ColumnDefinition/>
<ColumnDefinition/>
<ColumnDefinition/>
</Grid.ColumnDefinitions>
</Grid>
</ItemsPanelTemplate>
</ItemsControl.ItemsPanel>
<ItemsControl.ItemContainerStyle>
<Style TargetType="ContentPresenter">
<Setter Property="Grid.Row" Value="{Binding Row}"/>
<Setter Property="Grid.Column" Value="{Binding Column}"/>
</Style>
</ItemsControl.ItemContainerStyle>
</ItemsControl>
</Grid>
</Window>
Code Behind:
using System.Linq;
using System.Windows;
using System.ComponentModel;
using System.Collections.ObjectModel;
namespace MiscSamples
{
public partial class ChessBoard : Window
{
public ObservableCollection<ChessPiece> Pieces { get; set; }
public ChessBoard()
{
Pieces = new ObservableCollection<ChessPiece>();
InitializeComponent();
DataContext = Pieces;
NewGame();
}
private void NewGame()
{
Pieces.Clear();
Pieces.Add(new ChessPiece() { Row = 0, Column = 0, Type = ChessPieceTypes.Tower, IsBlack = true});
Pieces.Add(new ChessPiece() { Row = 0, Column = 1, Type = ChessPieceTypes.Knight, IsBlack = true });
Pieces.Add(new ChessPiece() { Row = 0, Column = 2, Type = ChessPieceTypes.Bishop, IsBlack = true });
Pieces.Add(new ChessPiece() { Row = 0, Column = 3, Type = ChessPieceTypes.Queen, IsBlack = true });
Pieces.Add(new ChessPiece() { Row = 0, Column = 4, Type = ChessPieceTypes.King, IsBlack = true });
Pieces.Add(new ChessPiece() { Row = 0, Column = 5, Type = ChessPieceTypes.Bishop, IsBlack = true });
Pieces.Add(new ChessPiece() { Row = 0, Column = 6, Type = ChessPieceTypes.Knight, IsBlack = true });
Pieces.Add(new ChessPiece() { Row = 0, Column = 7, Type = ChessPieceTypes.Tower, IsBlack = true });
Enumerable.Range(0, 8).Select(x => new ChessPiece()
{
Row = 1,
Column = x,
IsBlack = true,
Type = ChessPieceTypes.Pawn
}).ToList().ForEach(Pieces.Add);
Pieces.Add(new ChessPiece() { Row = 7, Column = 0, Type = ChessPieceTypes.Tower, IsBlack = false });
Pieces.Add(new ChessPiece() { Row = 7, Column = 1, Type = ChessPieceTypes.Knight, IsBlack = false });
Pieces.Add(new ChessPiece() { Row = 7, Column = 2, Type = ChessPieceTypes.Bishop, IsBlack = false });
Pieces.Add(new ChessPiece() { Row = 7, Column = 3, Type = ChessPieceTypes.Queen, IsBlack = false });
Pieces.Add(new ChessPiece() { Row = 7, Column = 4, Type = ChessPieceTypes.King, IsBlack = false });
Pieces.Add(new ChessPiece() { Row = 7, Column = 5, Type = ChessPieceTypes.Bishop, IsBlack = false });
Pieces.Add(new ChessPiece() { Row = 7, Column = 6, Type = ChessPieceTypes.Knight, IsBlack = false });
Pieces.Add(new ChessPiece() { Row = 7, Column = 7, Type = ChessPieceTypes.Tower, IsBlack = false });
Enumerable.Range(0, 8).Select(x => new ChessPiece()
{
Row = 6,
Column = x,
IsBlack = false,
Type = ChessPieceTypes.Pawn
}).ToList().ForEach(Pieces.Add);
}
}
ViewModel:
public class ChessPiece: INotifyPropertyChanged
{
public bool IsBlack { get; set; }
public ChessPieceTypes Type { get; set; }
private int _row;
public int Row
{
get { return _row; }
set
{
_row = value;
OnPropertyChanged("Row");
}
}
private int _column;
public int Column
{
get { return _column; }
set
{
_column = value;
OnPropertyChanged("Column");
}
}
public event PropertyChangedEventHandler PropertyChanged;
protected virtual void OnPropertyChanged(string propertyName)
{
PropertyChangedEventHandler handler = PropertyChanged;
if (handler != null) handler(this, new PropertyChangedEventArgs(propertyName));
}
public string ImageSource
{
get { return "../ChessPieces/" + (IsBlack ? "Black" : "White") + Type.ToString() + ".png"; }
}
}
public enum ChessPieceTypes
{
Pawn,
Tower,
Knight,
Bishop,
Queen,
King,
}
}
This is what it looks like in my computer:
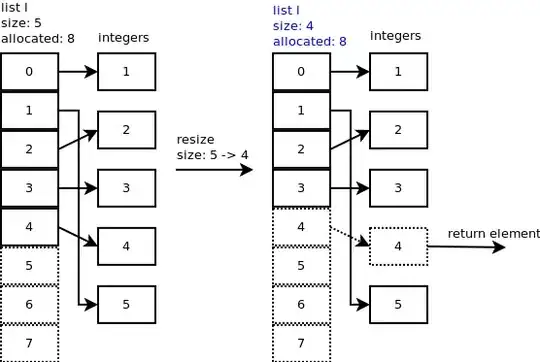
Please notice that I'm using pure XAML to create the UI. In no way Im creating nor manipulating UI elements in code. WPF doesn't need that, and it's also not recommended.
The recommended approach to WPF is to use MVVM and understand that UI is Not Data
You can copy and paste my code in a File -> New Project -> WPF Application
and see the results for yourself. You will need the following project structure:
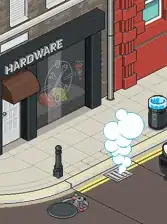
Also note that the Image files need to be set to Build Action: Resource
.
Remember: This is the WPF approach to EVERYTHING
. You rarely have to manipulate UI elements in code in WPF, or do things such as drawing or anything like that.