UIImage* backgroundImage = [UIImage imageNamed:kBackName];
CALayer* aLayer = [CALayer layer];
CGFloat nativeWidth = CGImageGetWidth(backgroundImage.CGImage);
CGFloat nativeHeight = CGImageGetHeight(backgroundImage.CGImage);
CGRect startFrame = CGRectMake(0.0, 0.0, nativeWidth, nativeHeight);
aLayer.contents = (id)backgroundImage.CGImage;
aLayer.frame = startFrame;
or in a Swift playground (you will have to provide your own PNG image in the Playground's resource file. I'm using the example of "FrogAvatar".)
//: Playground - noun: a place where people can play
import UIKit
if let backgroundImage = UIImage(named: "FrogAvatar") // you will have to provide your own image in your playground's Resource file
{
let height = backgroundImage.size.height
let width = backgroundImage.size.width
let aLayer = CALayer()
let startFrame = CGRect(x: 0, y: 0, width: width, height: height)
let aView = UIView(frame: startFrame)
aLayer.frame = startFrame
aLayer.contentsScale = aView.contentScaleFactor
aLayer.contents = backgroundImage.cgImage
aView.layer.addSublayer(aLayer)
aView // look at this via the Playground's eye icon
}
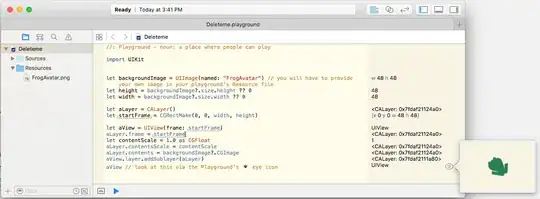