I've had time to put this together for you as an Excel formula. See my original comments at the foot of this posting for links to my sources.
Steps to resolve this:
User Defined Function to sort a cell
Create a new module in Excel VBA (let me know if you need instructions on this, it's only a couple of clicks)
Copy and paste all the code below.
Option Explicit
Const c_Separator = "/"
' User Defined Function to split a list within a cell and then sort it
' before recreating a sorted list
Public Function CellSort(strString As String) As String
Dim i As Integer
Dim arr As Variant
Dim strRet As String
arr = Split(strString, c_Separator)
' trim values so sort will work properly
For i = LBound(arr) To UBound(arr)
arr(i) = Trim(arr(i))
Next i
' sort
QuickSort arr, LBound(arr), UBound(arr)
' construct ordered list to return
For i = LBound(arr) To UBound(arr) - 1
strRet = strRet & CStr(arr(i)) & c_Separator
Next i
' Attach the last item separately to avoid adding an unecessary separator
CellSort = strRet & CStr(arr(i))
End Function
' Quick Sort function found here:
' https://stackoverflow.com/questions/3399823/excel-how-do-i-sort-within-a-cell
Public Sub QuickSort(vArray As Variant, inLow As Long, inHi As Long)
Dim pivot As Variant
Dim tmpSwap As Variant
Dim tmpLow As Long
Dim tmpHi As Long
tmpLow = inLow
tmpHi = inHi
pivot = vArray((inLow + inHi) \ 2)
While (tmpLow <= tmpHi)
While (vArray(tmpLow) < pivot And tmpLow < inHi)
tmpLow = tmpLow + 1
Wend
While (pivot < vArray(tmpHi) And tmpHi > inLow)
tmpHi = tmpHi - 1
Wend
If (tmpLow <= tmpHi) Then
tmpSwap = vArray(tmpLow)
vArray(tmpLow) = vArray(tmpHi)
vArray(tmpHi) = tmpSwap
tmpLow = tmpLow + 1
tmpHi = tmpHi - 1
End If
Wend
If (inLow < tmpHi) Then QuickSort vArray, inLow, tmpHi
If (tmpLow < inHi) Then QuickSort vArray, tmpLow, inHi
End Sub
Close the VBA editor (no more coding required).
Excel Formulas to Calculate number of Unique combinations
In Excel you create three columns alongside your original column of data. I have shown these formulas in the screenshot below in the columns with coloured backgrounds. Each is explained beneath the image.
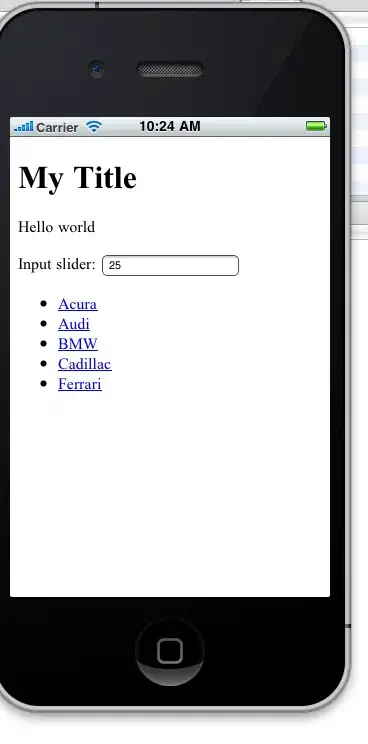
Blue column: Uses the VBA function above to sort the contents of each cell in the original list, this gives a consistent list that you can count unique items on. If your original list has some instances in lowercase and others in uppercase and you need to treat these as the same then modify the formula in this column to be =CellSort(UPPER(A2))
Green column: Simple COUNTIF
function (works in all recent Excel versions) that identifies the first instance of each sorted cell.
Red cell: Counts the number of times TRUE appears in the green column. This gives a count of unique entries.
And below is an example of the completed work.
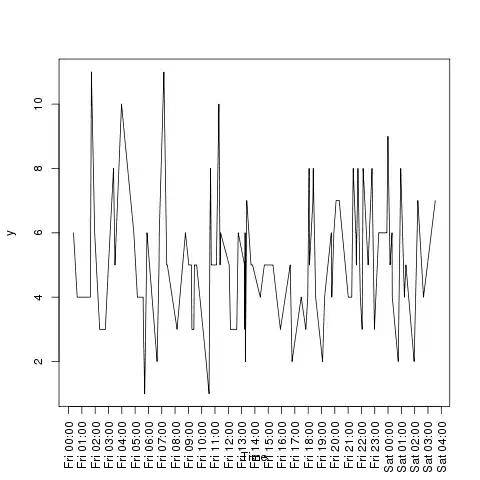
Original comments
Afraid I don't have time to test this right now but this may help you.
You might want to look at the VBA given in this answer (I haven't tried it myself).
VBA QuickSort
Then, if you need to do this in formulas, you could create a User Defined Function from this VBA to sort the values in the cell. Change the line arr = Split(ActiveCell.Text, ",")
to have "/"
so it will split your list.
Next use your formula in a column alongside the original data and then use something like the formulas on this page: Count occurrences of values or unique values in a data range to count unique.
Let me know if you need more help with any of the above and I will try to do it tomorrow.