Sigh, in Windows Forms it was literally one line. In WPF it looks like it will be several hundred.
That's because it was the ONLY thing you could place in a button in winforms.
WPF is a true UI framework, not some random semi-deprecated dinosaur that only allows to do the default stuff (which by the way looks horrible).
Option 1: Place the Image as Button's Content
:
If you just want to place an Image in the button, and nothing else, why not just do that?
<Style TargetType="Button">
<Setter Property="Background" Value="LimeGreen"/>
<Setter Property="Template">
<Setter.Value>
<ControlTemplate TargetType="Button">
<Border Background="{TemplateBinding Background}">
<ContentPresenter ContentSource="Content"/>
</Border>
</ControlTemplate>
</Setter.Value>
</Setter>
</Style>
Then:
<Button>
<!-- Background not set, defaults to what's set in the Style -->
<Image Source="./ChessPieces/BlackKnight.png"/>
</Button>
<Button Background="Red">
<Image Source="./ChessPieces/BlackBishop.png"/>
</Button>
<Button Background="Blue">
<Image Source="./ChessPieces/BlackPawn.png"/>
</Button>
<!-- WPF's idea of "Dynamic" is not the same as win(hack)forms' -->
<Button Background="White">
<Image Source="{Binding SomeStringPropertyDefinedInAViewModel}"/>
</Button>
Result:

Option 2 (not very elegant): Use the Tag
Property:
If, in addition to the Image, you want to put something else inside the Button, you can resort to a somewhat hacky approach of putting the ImageSource in the Button's Tag
property.
<Style TargetType="Button">
<Setter Property="Background" Value="LimeGreen"/>
<Setter Property="Template">
<Setter.Value>
<ControlTemplate TargetType="Button">
<Border Background="{TemplateBinding Background}">
<StackPanel Orientation="Horizontal">
<Image Source="{Binding Tag, RelativeSource={RelativeSource TemplatedParent}}"
Height="50" Width="50"/>
<ContentPresenter ContentSource="Content"/>
</StackPanel>
</Border>
</ControlTemplate>
</Setter.Value>
</Setter>
</Style>
Then:
<Button Tag="./ChessPieces/BlackKnight.png"
Background="Blue"
Foreground="White"
Content="Some Text"/>
<Button Tag="./ChessPieces/BlackBishop.png"
Background="LightGray">
<CheckBox Content="A CheckBox!" VerticalAlignment="Center"/>
</Button>
Result:
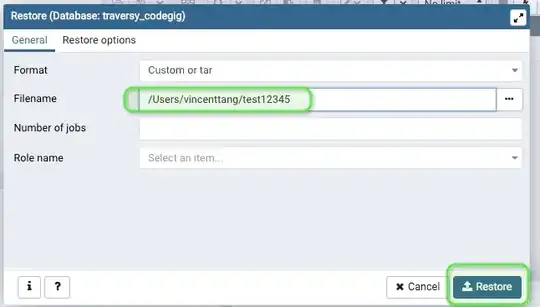
Option 3: Use an Attached Property
Same as option 2, but using a property declared elsewhere, for example:
<Button ButtonImage.Source="./ChessPieces/BlackBishop.png"
<!-- etc -->
Option 4: Create a Custom Control:
Create a class (.cs file with no XAML) derived from Button and add a DependencyProperty
to hold the image, then set the template to that and use that value.
Option 5: MVVM
Create a ButtonViewModel
, or actually use a DelegateCommand declared in the ViewModel to bind the Button's properties.
Not an Option: Traverse the Visual Tree and change the Image.Source
in code.
That's not something you will want to do. It's not a good approach at all.
I could go on forever, but I have to go to sleep. If you want me to elaborate on any of these approaches just let me know.