Using block:
using System;
using System.Net;
using System.Net.Http;
This Function will create new HttpClient object, set http-method to GET, set request URL to the function "Url" string argument and apply these parameters to HttpRequestMessage object (which defines settings of SendAsync method). Last line: function sends async GET http request to the specified url, waits for response-message's .Result property(just full response object: headers + body/content), gets .Content property of that full response(body of request, without http headers), applies ReadAsStringAsync() method to that content(which is also object of some special type) and, finally, wait for this async task to complete using .Result property once again in order to get final result string and then return this string as our function return.
static string GetHttpContentAsString(string Url)
{
HttpClient HttpClient = new HttpClient();
HttpRequestMessage RequestMessage = new HttpRequestMessage(HttpMethod.Get, Url);
return HttpClient.SendAsync(RequestMessage).Result.Content.ReadAsStringAsync().Result;
}
Shorter version, which does not show the full "transformational" path of our http-request and uses GetStringAsync method of HttpClient object. Function just creates new instance of HttpClient class (an HttpClient object), uses GetStringAsync method to get response body(content) of our http request as an async-task result\promise, and then uses .Result property of that async-task-result to get final string and after that simply returns this string as a function return.
static string GetStringSync(string Url)
{
HttpClient HttpClient = new HttpClient();
return HttpClient.GetStringAsync(Url).Result;
}
Usage:
const string url1 = "https://microsoft.com";
const string url2 = "https://stackoverflow.com";
ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls12; /*sets TLC protocol version explicitly to modern version, otherwise C# could not make http requests to some httpS sites, such as https://microsoft.com*/
Console.WriteLine(GetHttpContentAsString(url1)); /*gets microsoft main page html*/
Console.ReadLine(); /*makes some pause before second request. press enter to make second request*/
Console.WriteLine(GetStringSync(url2)); /*gets stackoverflow main page html*/
Console.ReadLine(); /*press enter to finish*/
Full code:
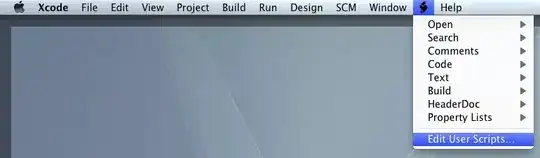