this value isn't possible to override by using UIManager for JTabbedPane,
this is quite common issue that most of methods are protected (doesn't matter if they are published as keys in UIManager) without overriding own BasicTabbedPaneUI
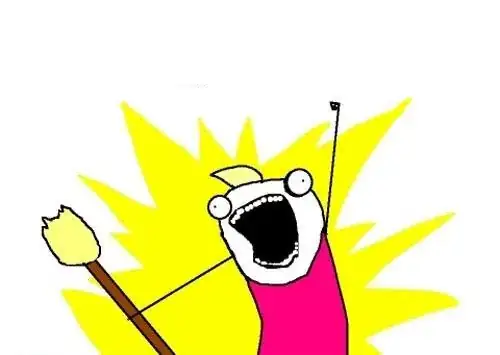
javax.swing.plaf.InsetsUIResource[top=4,left=4,bottom=4,right=4]
false

java.awt.Insets[top=4,left=2,bottom=3,right=3]
false
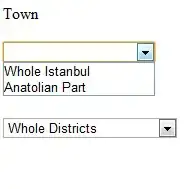
null
null
system (win8 on Java6)
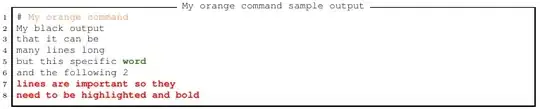
javax.swing.plaf.InsetsUIResource[top=2,left=2,bottom=3,right=3]
true
- doesn't matter if value for key is in form
Instets(0,0,0,0)
or InsetsUIResource(0,0,0,0)
modified code originnaly made by Kirill
import java.awt.BorderLayout;
import java.awt.FlowLayout;
import java.awt.Insets;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.*;
import org.pushingpixels.substance.api.DecorationAreaType;
import org.pushingpixels.substance.api.SubstanceLookAndFeel;
import org.pushingpixels.substance.api.skin.BusinessBlueSteelSkin;
import org.pushingpixels.substance.api.skin.OfficeBlack2007Skin;
import org.pushingpixels.substance.api.skin.OfficeBlue2007Skin;
/**
* Test application that shows the use of the
* {@link SubstanceLookAndFeel#getDecorationType(java.awt.Component)} API called
* on different components.
*
* @author Kirill Grouchnikov
* @see SubstanceLookAndFeel#getDecorationType(java.awt.Component)
*/
public class GetDecorationType /*extends JFrame*/ {
private static final long serialVersionUID = 1L;
private static JFrame frame = new JFrame();
/**
* Creates the main frame for
* <code>this</code> sample.
*/
public GetDecorationType() {
frame.setTitle("Get decoration type");
frame.setLayout(new BorderLayout());
final JTabbedPane tabs = new JTabbedPane();
SubstanceLookAndFeel.setDecorationType(tabs, DecorationAreaType.HEADER);
JPanel tab1 = new JPanel(new FlowLayout());
tab1.add(new JTextField("sample"));
final JComboBox combo = new JComboBox(new Object[]{"sample"});
tab1.add(combo);
SubstanceLookAndFeel.setDecorationType(tab1, DecorationAreaType.GENERAL);
JPanel tab2 = new JPanel(new FlowLayout());
tab2.add(new JTextField("sample2"));
tab2.add(new JComboBox(new Object[]{"sample2"}));
SubstanceLookAndFeel.setDecorationType(tab2, DecorationAreaType.GENERAL);
tabs.addTab("tab1", tab1);
tabs.addTab("tab2", tab2);
frame.add(tabs, BorderLayout.CENTER);
JPanel controlPanel = new JPanel(new FlowLayout(FlowLayout.RIGHT));
JButton getTypes = new JButton("Get types");
getTypes.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
DecorationAreaType tabsType = SubstanceLookAndFeel.getDecorationType(tabs);
DecorationAreaType comboType = SubstanceLookAndFeel.getDecorationType(combo);
JOptionPane.showMessageDialog(frame, "Tabbed pane: " + tabsType.getDisplayName()
+ "\n" + "Combo box: " + comboType.getDisplayName());
}
});
}
});
controlPanel.add(getTypes);
frame.add(controlPanel, BorderLayout.SOUTH);
frame.setSize(400, 200);
frame.setLocationRelativeTo(null);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
/**
* The main method for
* <code>this</code> sample. The arguments are ignored.
*
* @param args Ignored.
*/
public static void main(String[] args) {
JFrame.setDefaultLookAndFeelDecorated(true);
JDialog.setDefaultLookAndFeelDecorated(true);
try {
for (UIManager.LookAndFeelInfo info : UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
UIManager.setLookAndFeel(info.getClassName());
break;
}
}
/*UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());*/
} catch (Exception e) {
return;
}
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
//SubstanceLookAndFeel.setSkin(new BusinessBlueSteelSkin());
//SubstanceLookAndFeel.setSkin(new OfficeBlack2007Skin());
SubstanceLookAndFeel.setSkin(new OfficeBlue2007Skin());
UIManager.put("TabbedPane.contentOpaque", Boolean.TRUE);
System.out.println(UIManager.getDefaults().get("TabbedPane.contentBorderInsets"));
System.out.println(UIManager.getDefaults().get("TabbedPane.tabsOverlapBorder"));
UIManager.getDefaults().put("TabbedPane.contentBorderInsets", new javax.swing.plaf.InsetsUIResource(0, 0, 0, 0));
UIManager.getDefaults().put("TabbedPane.tabsOverlapBorder", true);
SwingUtilities.updateComponentTreeUI(frame);
new GetDecorationType()/*.setVisible(true)*/;
}
});
}
}