Based on Ahmed Nuaman answer (please also give them +1), I have prepared this piece of code:
example of usage:
To analyze most active facebook users of http://www.facebook.com/cern
$ python FacebookFanAnalyzer.py cern likes
$ python FacebookFanAnalyzer.py cern comments
$ python FacebookFanAnalyzer.py cern likes comments
notes: shares and inner comments are not supported
file: FacebookFanAnalyzer.py
# -*- coding: utf-8 -*-
import json
import urllib2
import sys
from collections import Counter
reload(sys)
sys.setdefaultencoding('utf8')
###############################################################
###############################################################
#### PLEASE PASTE HERE YOUR TOKEN, YOU CAN GENERETE IT ON:
#### https://developers.facebook.com/tools/explorer
#### GENERETE AND PASTE NEW ONE, WHEN THIS WILL STOP WORKING
token = 'AjZCBe5yhAq2zFtyNS4tdPyhAq2zFtyNS4tdPw9sMkSUgBzF4tdPw9sMkSUgBzFZCDcd6asBpPndjhAq2zFtyNS4tsBphqfZBJNzx'
attrib_limit = 100
post_limit = 100
###############################################################
###############################################################
class FacebookFanAnalyzer(object):
def __init__(self, fanpage_name, post_limit, attribs, attrib_limit):
self.fanpage_name = fanpage_name
self.post_limit = post_limit
self.attribs = attribs
self.attrib_limit = attrib_limit
self.data={}
def make_request(self, attrib):
global token
url = 'https://graph.facebook.com/' + self.fanpage_name + '/posts?limit=' + str(self.post_limit) + '&fields=' + attrib + '.limit('+str(self.attrib_limit)+')&access_token=' + token
print "Requesting '" + attrib + "' data: " + url
req = urllib2.urlopen(url)
res = json.loads(req.read())
if res.get('error'):
print res['error']
exit()
return res
def grep_data(self, attrib):
res=self.make_request(attrib)
lst=[]
for status in res['data']:
if status.get(attrib):
for person in status[attrib]['data']:
if attrib == 'likes':
lst.append(person['name'])
elif attrib == 'comments':
lst.append(person['from']['name'])
return lst
def save_as_html(self, attribs):
filename = self.fanpage_name + '.html'
f = open(filename, 'w')
f.write(u'<html><head></head><body>')
f.write(u'<table border="0"><tr>')
for attrib in attribs:
f.write(u'<td>'+attrib+'</td>')
f.write(u'</tr>')
for attrib in attribs:
f.write(u'<td valign="top"><table border="1">')
for d in self.data[attrib]:
f.write(u'<tr><td>' + unicode(d[0]) + u'</td><td>' +unicode(d[1]) + u'</td></tr>')
f.write(u'</table></td>')
f.write(u'</tr></table>')
f.write(u'</body>')
f.close()
print "Saved to " + filename
def fetch_data(self, attribs):
for attrib in attribs:
self.data[attrib]=Counter(self.grep_data(attrib)).most_common()
def main():
global post_limit
global attrib_limit
fanpage_name = sys.argv[1]
attribs = sys.argv[2:]
f = FacebookFanAnalyzer(fanpage_name, post_limit, attribs, attrib_limit)
f.fetch_data(attribs)
f.save_as_html(attribs)
if __name__ == '__main__':
main()
Output:
Requesting 'comments' data: https://graph.facebook.com/cern/posts?limit=50&fields=comments.limit(50)&access_token=AjZCBe5yhAq2zFtyNS4tdPyhAq2zFtyNS4tdPw9sMkSUgBzF4tdPw9sMkSUgBzFZCDcd6asBpPndjhAq2zFtyNS4tsBphqfZBJNzx
Requesting 'likes' data: https://graph.facebook.com/cern/posts?limit=50&fields=likes.limit(50)&access_token=AjZCBe5yhAq2zFtyNS4tdPyhAq2zFtyNS4tdPw9sMkSUgBzF4tdPw9sMkSUgBzFZCDcd6asBpPndjhAq2zFtyNS4tsBphqfZBJNzx
Saved to cern.html
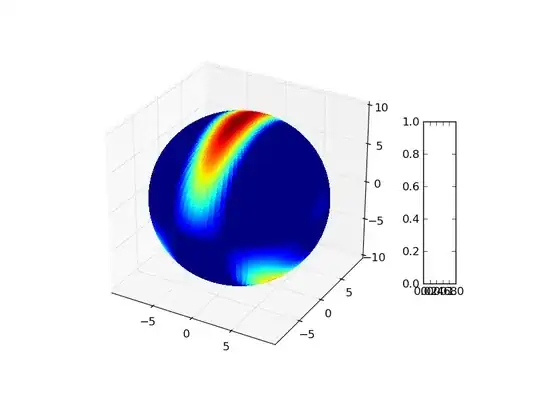