This Explanation is the basic reason behind a StackOverflowException for Java, C, and C++.
A stack overflow exception is generally caused in any language due to recursive method calls.
Suppose you have an method which is calling itself or any other method for an infinite, recursive loop; it will cause a Stackoverflowexception. The reason behind this is the method call stack gets filled, and it won't be able to accommodate any other method call.
Method call stack looks like this picture.
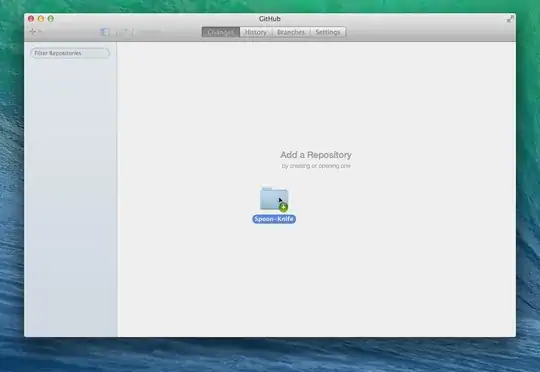
Explanation -- Suppose the Main method has five statements, and the third method has an call to methodA, then the execution of Main method gets paused at statement3 and methodA will be loaded into the call stack. Then method A has a call to methodB. So methodB also gets loaded into the stack.
So in this way infinite, recursive calls make the call stack get filled at which point it can't afford any more methods. So it throws a StackOverflowException.