The best solution is to put your control (the image) into a window (control) that handles the dialog keys, and then to make sure that this control receives keyboard focus at the appropriate times.
Well, honestly, you seem to draw/animate things by moving graphic controls. The very best way is to create a custom control (descending from TCustomControl
) and draw things manually (or, possibly, to have graphic child controls). Then you can easily make this control respond to the arrow keys.
This control does it:
unit WindowContainer;
interface
uses
SysUtils, Windows, Messages, Classes, Controls;
type
TWindowContainer = class(TCustomControl)
private
protected
procedure WMGetDlgCode(var Message: TWMGetDlgCode); message WM_GETDLGCODE;
public
constructor Create(AOwner: TComponent); override;
published
property OnStartDrag;
property OnStartDock;
property OnUnDock;
property OnClick;
property OnCanResize;
property OnAlignPosition;
property OnAlignInsertBefore;
property OnResize;
property OnMouseWheel;
property OnMouseWheelDown;
property OnMouseWheelUp;
property OnMouseUp;
property OnMouseMove;
property OnMouseLeave;
property OnMouseEnter;
property OnMouseDown;
property OnMouseActivate;
property OnKeyUp;
property OnKeyPress;
property OnKeyDown;
end;
procedure Register;
implementation
procedure Register;
begin
RegisterComponents('Rejbrand 2009', [TWindowContainer]);
end;
{ TWindowContainer }
constructor TWindowContainer.Create(AOwner: TComponent);
begin
inherited;
ControlStyle := [csAcceptsControls, csCaptureMouse, csClickEvents,
csSetCaption, csDoubleClicks, csReplicatable, csPannable,
csFramed];
end;
procedure TWindowContainer.WMGetDlgCode(var Message: TWMGetDlgCode);
begin
inherited;
Message.Result := Message.Result or DLGC_WANTCHARS or DLGC_WANTARROWS
or DLGC_WANTTAB or DLGC_WANTALLKEYS;
end;
end.
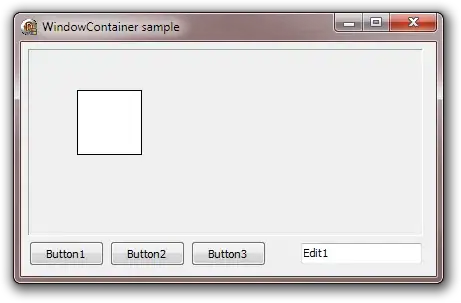
Sample demo EXE
To try this control, simply put the shape/image in it, and use the OnKeyDown
event of this control. Also, make sure to set focus to this control, e.g. when the user clicks it:
procedure TForm1.WindowContainer1Click(Sender: TObject);
begin
WindowContainer1.SetFocus;
end;
Now, doing animations by moving controls is bad. Instead, you should draw manually. Combine this answer with the code in this answer and you're done.