LINQ is for querying collection, For modification your current loop is more readable and better approach, but if you want LINQ option then:
If OrderInfo
is a class (reference type), then you can modify the properties of the object, (You can't assign them null or a new references).
var ordersList = new List<OrdersInfo>();
ordersList.Add(new OrdersInfo() { TYPE = "P" });
ordersList.Add(new OrdersInfo() { TYPE = "S" });
ordersList.Add(new OrdersInfo() { TYPE = "P" });
ordersList.Select(r => (r.TYPE == "P" ? r.TYPE = "Project" : r.TYPE = "Support")).ToList();
With Your class defined as:
class OrdersInfo
{
public string TYPE { get; set; }
}
Here is the screenshot
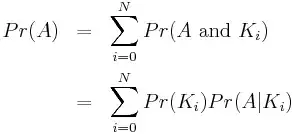
Interestingly I didn't assign the result back to ordersList