PUSH WITHOUT ANIMATION : Swift Here is what worked for me.
import ObjectiveC
private var AssociatedObjectHandle: UInt8 = 0
extension UIViewController {
var isAnimationRequired:Bool {
get {
return (objc_getAssociatedObject(self, &AssociatedObjectHandle) as? Bool) ?? true
}
set {
objc_setAssociatedObject(self, &AssociatedObjectHandle, newValue, objc_AssociationPolicy.OBJC_ASSOCIATION_RETAIN_NONATOMIC)
}
}
}
-------------------- SilencePushSegue --------------------
class SilencePushSegue: UIStoryboardSegue {
override func perform() {
if self.source.isAnimationRequired == false {
self.source.navigationController?.pushViewController(self.destination, animated: false)
}else{
self.source.navigationController?.pushViewController(self.destination, animated: true)
}
}
}
Usage : Set the segue class from storyboard as shown in picture. set the isAnimationRequired from your viewcontroller to false from where you want to call performSegue, when you want to push segue without animation and set back to true after calling self.performSegue. Best of luck....
DispatchQueue.main.async {
self.isAnimationRequired = false
self.performSegue(withIdentifier: "showAllOrders", sender: self);
self.isAnimationRequired = true
}
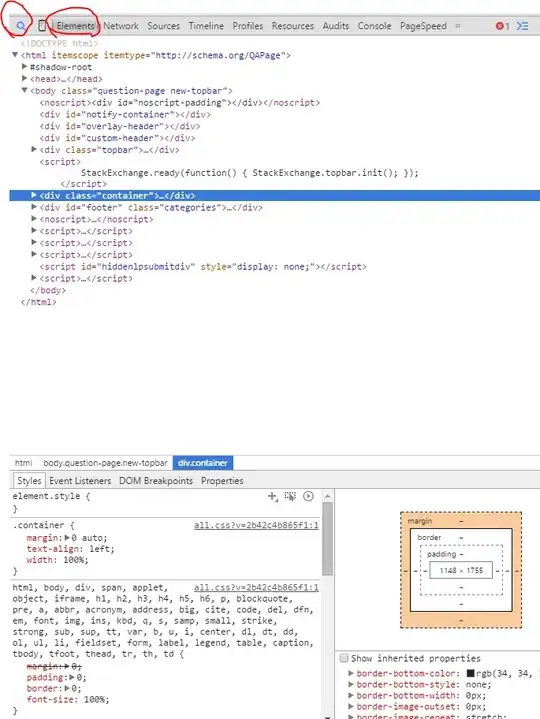