With the help of a book, Swing Hacks, I created a sliding JFrame and a sliding JDialog. It took me a few hours to debug the code.
Here's a test run showing a JOptionPane slid part way out.
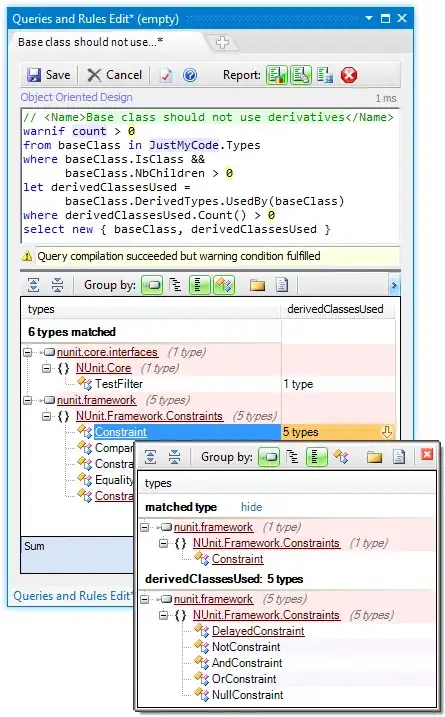
And all the way out.
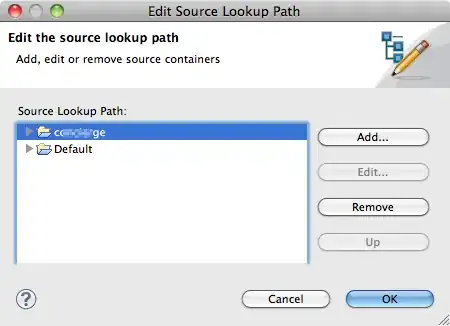
Basically, I took the content pane of the JOptionPane, and animated it as an image.
You left click the slide sheet button to slide the JOptionPane out. You left click Yes or No on the JOptionPane to slide the JOptionPane back.
Here's the code to create the animated JFrame and animated JDialog.
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.GraphicsConfiguration;
import java.awt.GraphicsEnvironment;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.image.BufferedImage;
import javax.swing.Box;
import javax.swing.JComponent;
import javax.swing.JDialog;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.Timer;
import javax.swing.border.LineBorder;
public class AnimatedJFrame extends JFrame implements ActionListener {
private static final long serialVersionUID = 6462856212447879086L;
public static final int INCOMING = 1;
public static final int OUTGOING = -1;
public static final float ANIMATION_DURATION = 600F;
public static final int ANIMATION_SLEEP = 5;
JComponent sheet;
JPanel glass;
AnimatingSheet animatingSheet;
boolean animating;
int animationDirection;
Timer animationTimer;
long animationStart;
BufferedImage offscreenImage;
public AnimatedJFrame(String name) {
super(name);
glass = (JPanel) getGlassPane();
glass.setLayout(new GridBagLayout());
animatingSheet = new AnimatingSheet();
animatingSheet.setBorder(new LineBorder(Color.BLACK, 1));
}
public JComponent showJDialogAsSheet(JDialog dialog) {
sheet = (JComponent) dialog.getContentPane();
sheet.setBorder(new LineBorder(Color.BLACK, 1));
glass.removeAll();
animationDirection = INCOMING;
startAnimation();
return sheet;
}
public void hideSheet() {
animationDirection = OUTGOING;
startAnimation();
}
private void startAnimation() {
// glass.repaint();
// Clear glass pane and set up animatingSheet
animatingSheet.setSource(sheet);
glass.removeAll();
setGridBagConstraints(animatingSheet);
glass.setVisible(true);
// Start animation timer
animationStart = System.currentTimeMillis();
if (animationTimer == null) {
animationTimer = new Timer(ANIMATION_SLEEP, this);
}
animating = true;
animationTimer.start();
}
private void stopAnimation() {
animationTimer.stop();
animating = false;
}
@Override
public void actionPerformed(ActionEvent event) {
if (animating) {
// Calculate height to show
float animationPercent = (System.currentTimeMillis() - animationStart)
/ ANIMATION_DURATION;
animationPercent = Math.min(1.0F, animationPercent);
int animatingWidth = 0;
if (animationDirection == INCOMING) {
animatingWidth = (int) (animationPercent * sheet.getWidth());
} else {
animatingWidth = (int) ((1.0F - animationPercent) * sheet
.getWidth());
}
// Clip off that much from the sheet and blit it
// into the animatingSheet
animatingSheet.setAnimatingWidth(animatingWidth);
animatingSheet.repaint();
if (animationPercent >= 1.0F) {
stopAnimation();
if (animationDirection == INCOMING) {
finishShowingSheet();
} else {
glass.removeAll();
glass.setVisible(false);
}
}
}
}
private void finishShowingSheet() {
glass.removeAll();
setGridBagConstraints(sheet);
glass.revalidate();
glass.repaint();
}
private void setGridBagConstraints(JComponent sheet) {
GridBagConstraints gbc = new GridBagConstraints();
gbc.anchor = GridBagConstraints.NORTHWEST;
glass.add(sheet, gbc);
gbc.gridy = 1;
gbc.weighty = Integer.MAX_VALUE;
glass.add(Box.createGlue(), gbc);
}
}
class AnimatingSheet extends JPanel {
private static final long serialVersionUID = 3958155417286820827L;
Dimension animatingSize = new Dimension(0, 1);
JComponent source;
BufferedImage offscreenImage;
public AnimatingSheet() {
super();
setOpaque(true);
}
public void setSource(JComponent source) {
this.source = source;
animatingSize.height = source.getHeight();
makeOffscreenImage(source);
}
public void setAnimatingWidth(int width) {
animatingSize.width = width;
setSize(animatingSize);
}
private void makeOffscreenImage(JComponent source) {
GraphicsConfiguration gfxConfig = GraphicsEnvironment
.getLocalGraphicsEnvironment().getDefaultScreenDevice()
.getDefaultConfiguration();
offscreenImage = gfxConfig.createCompatibleImage(source.getWidth(),
source.getHeight());
Graphics2D offscreenGraphics = (Graphics2D) offscreenImage
.getGraphics();
source.paint(offscreenGraphics);
offscreenGraphics.dispose();
}
@Override
public Dimension getPreferredSize() {
return animatingSize;
}
@Override
public Dimension getMinimumSize() {
return animatingSize;
}
@Override
public Dimension getMaximumSize() {
return animatingSize;
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
BufferedImage fragment = offscreenImage.getSubimage(
offscreenImage.getWidth() - animatingSize.width, 0,
animatingSize.width, source.getHeight());
g.drawImage(fragment, 0, 0, this);
}
}
Here's the code to test the animated JFrame.
import java.awt.BorderLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JDialog;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.SwingUtilities;
public class SheetTest implements PropertyChangeListener, Runnable {
JOptionPane optionPane;
AnimatedJFrame frame;
public static void main(String[] args) {
SwingUtilities.invokeLater(new SheetTest());
}
@Override
public void run() {
// Build JOptionPane dialog
optionPane = new JOptionPane("Do you want to save?",
JOptionPane.QUESTION_MESSAGE, JOptionPane.YES_NO_OPTION);
optionPane.addPropertyChangeListener(this);
frame = new AnimatedJFrame("Sheet Test");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Put an image in the frame's content pane
ImageIcon icon = new ImageIcon("images/Google Tile.jpg");
JLabel label = new JLabel(icon);
frame.getContentPane().add(label, BorderLayout.CENTER);
JButton dropButton = new JButton("Slide sheet");
dropButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent event) {
JDialog dialog = optionPane.createDialog(frame, "Irrelevant");
frame.showJDialogAsSheet(dialog);
}
});
frame.getContentPane().add(dropButton, BorderLayout.SOUTH);
frame.pack();
frame.setVisible(true);
}
@Override
public void propertyChange(PropertyChangeEvent event) {
if (event.getPropertyName().equals(JOptionPane.VALUE_PROPERTY)) {
frame.hideSheet();
}
}
}
And here's the image I used to create the test JFrame.
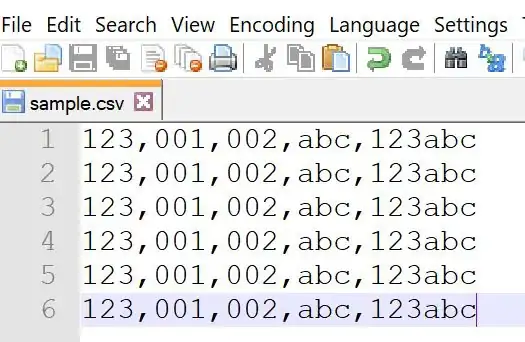