First of all, you must leave behind the traditional mentality of manipulating UI elements in code and Embrace MVVM
<Window x:Class="MiscSamples.QueryBuilderSample"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:MiscSamples"
Title="QueryBuilderSample" Height="300" Width="300">
<ItemsControl ItemsSource="{Binding}">
<ItemsControl.ItemTemplate>
<DataTemplate>
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition/>
<ColumnDefinition/>
<ColumnDefinition Width="Auto"/>
</Grid.ColumnDefinitions>
<ComboBox ItemsSource="{Binding Operators}"
SelectedItem="{Binding Operator}"/>
<TextBox Text="{Binding Value}" Grid.Column="1"/>
<Button Content="Add" Grid.Column="2"/>
</Grid>
</DataTemplate>
</ItemsControl.ItemTemplate>
</ItemsControl>
</Window>
Code behind:
public partial class QueryBuilderSample : Window
{
public List<QueryCriteria> Criterias { get; set; }
public QueryBuilderSample()
{
InitializeComponent();
DataContext = Criterias = Enumerable.Range(0, 10).Select(x => new QueryCriteria()).ToList();
}
}
ViewModel:
public class QueryCriteria
{
public List<Operators> Operators
{
get
{
return Enum.GetValues(typeof(Operators))
.Cast<Operators>()
.ToList();
}
}
public Operators Operator { get; set; }
public string Value { get; set; }
}
public enum Operators
{
Equals,
Contains,
GreaterThan,
SmallerThan,
}
Result:
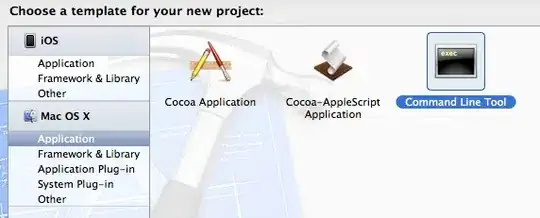
- Notice that I'm not doing a single line of code to create / manipulate UI elements. Everything is done via DataBinding.
- Simple code. No complex event handling stuff or anything like that.
- Declarative Code. Just Simple, Simple Properties and
INotifyPropertyChanged
. That's the default approach to EVERYTHING in WPF.