Example of amueller's code in action
In command-line / terminal:
sudo pip install wordcloud
Then run python script:
## Simple WordCloud
import matplotlib.pyplot as plt
from wordcloud import WordCloud, STOPWORDS
text = 'all your base are belong to us all of your base base base'
def generate_wordcloud(text): # optionally add: stopwords=STOPWORDS and change the arg below
wordcloud = WordCloud(font_path='/Library/Fonts/Verdana.ttf',
width=800, height=400,
relative_scaling = 1.0,
stopwords = {'to', 'of'} # set or space-separated string
).generate(text)
fig = plt.figure(1, figsize=(8, 4))
plt.axis('off')
plt.imshow(wordcloud)
plt.axis("off")
## Pick One:
# plt.show()
plt.savefig("WordCloud.png")
generate_wordcloud(text)
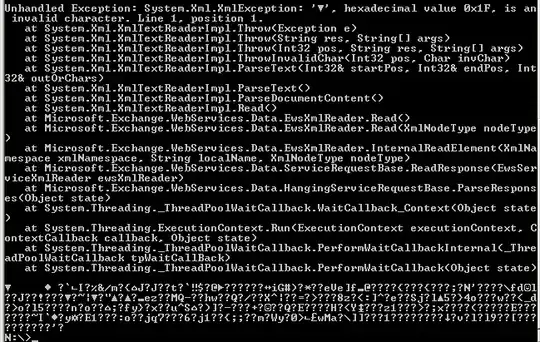