Ok. Delete all your code and start all over.
First of all, you have a SERIOUS misconception here: The UI is NOT the right place to store data.
Therefore, you should NOT be placing your numeric values in XAML, but instead you should create a proper ViewModel to store these numbers and operate on them:
<Window x:Class="MiscSamples.AverageNumbersSample"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="AverageNumbersSample" Height="300" Width="300">
<DockPanel>
<Button Content="Calculate" Click="Calculate" DockPanel.Dock="Top"/>
<ListBox ItemsSource="{Binding}">
<ListBox.ItemTemplate>
<DataTemplate>
<TextBlock Text="{Binding Value}" x:Name="txt"/>
<DataTemplate.Triggers>
<DataTrigger Binding="{Binding IsBelowAverage}" Value="True">
<Setter TargetName="txt" Property="Foreground" Value="Blue"/>
</DataTrigger>
</DataTemplate.Triggers>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
</DockPanel>
</Window>
Code Behind:
public partial class AverageNumbersSample : Window
{
public double Average = 116.21428571428571;
public List<AverageSampleViewModel> Values { get; set; }
public AverageNumbersSample()
{
InitializeComponent();
DataContext = Values = Enumerable.Range(100, 150)
.Select(x => new AverageSampleViewModel() { Value = x })
.ToList();
}
private void Calculate(object sender, RoutedEventArgs e)
{
Values.ForEach(x => x.IsBelowAverage = x.Value < Average);
}
}
Data Item:
public class AverageSampleViewModel: PropertyChangedBase
{
public int Value { get; set; }
private bool _isBelowAverage;
public bool IsBelowAverage
{
get { return _isBelowAverage; }
set
{
_isBelowAverage = value;
OnPropertyChanged("IsBelowAverage");
}
}
}
PropertyChangedBase class (MVVM Helper)
public class PropertyChangedBase:INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
protected virtual void OnPropertyChanged(string propertyName)
{
Application.Current.Dispatcher.BeginInvoke((Action) (() =>
{
PropertyChangedEventHandler handler = PropertyChanged;
if (handler != null) handler(this, new PropertyChangedEventArgs(propertyName));
}));
}
}
Result:
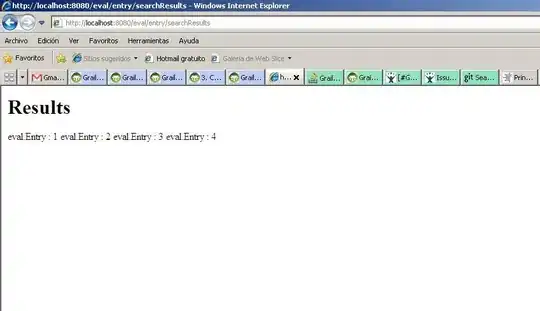
- In this sense, WPF is fundamentally different from anything else currently in existence (such as the multiple dinosaur archaic UI frameworks available for java or stuff like that).
- You must NEVER use the UI to
store
data, instead you use the UI to show
data. These concepts are fundamentally different.
- My example (which is the RIGHT way to do WPF applications), removes the need for
int.Parse()
or any casting stuff like that, because the ViewModel already has the proper data type required.