java.time.ZonedDateTime
The Calendar
class is terrible, as is its usual concrete class, GregorianCalendar
. These legacy classes were years ago supplanted by the modern java.time classes, specifically ZonedDateTime
.
When encountering a Calendar
object, see if it is indeed a GregorianCalendar
object. If so, convert using new to…
/from…
methods added to the old classes.
if( myCal instanceof GregorianCalendar ) {
GregorianCalendar gc = ( GregorianCalendar ) myCal ; // Cast to the concrete type.
ZonedDateTime zdt = gc.toZonedDateTime() ; // Convert from legacy class to modern class.
}
Immutable objects
The java.time classes wisely use the immutable objects pattern, appropriate to their purpose.
So you do not manipulate individual member fields within an existing object. Instead, you pass a new field value to produce a new object based on the combination of original values and your specific different value. See the with
methods.
Adding/subtracting a span of time
In your case, it seems you want to perform addition or subtraction to arrive at a new moment.
Specify a span of time by using:
Duration
for a scale of hours-minutes-seconds
Period
for a scale of years-months-days.
Example: Add a few days to get a moment later.
Period p = Period.ofDays( 3 ) ;
ZonedDateTime threeDaysLater = zdt.plus( p ) ;
For doing this kind of work, you might find handy the Interval
and LocalDateRange
classes offered by the ThreeTen-Extra library linked below.
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes.
Where to obtain the java.time classes?
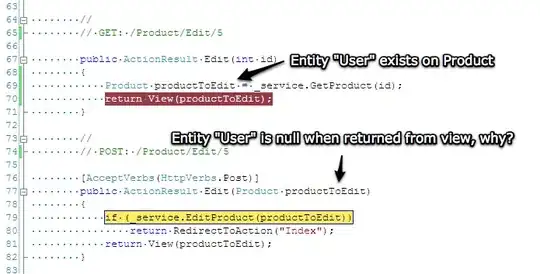
The ThreeTen-Extra project extends java.time with additional classes. This project is a proving ground for possible future additions to java.time. You may find some useful classes here such as Interval
, YearWeek
, YearQuarter
, and more.