You can access U2 Database (UniData or UniVerse) using U2 Toolkit for .NET the following ways:
- SQL Access (UCI Server)
- Native Access (UO Server)
SQL Access
For SQL Access, you need to normalize U2 Account (getting schema). For this, you can use the following tools:
- HS.ADMIN (for UniVerse Database) (http://www.rocketsoftware.com/u2/products/u2-clients-and-db-tools/u2-resources/universe-11.1-clients/copy_of_uvodbc-v11r1.pdf/view)
- VSG (for UniData Database)
- MDM ( for UniVerse Database and UniData Database)
You can use U2 Toolkit for .NET’s U2 Database Provider for .NET (ADO.NET Provider) for SQL access
Native Access
For Native Access, you do not need to do anything.
You can use U2 Toolkit for .NET’s UO API for Native Access.
Sample Code and MSDN Style Help
There are tons of sample code on SQL Access and Native Access when you install the product.
The best thing for you is to install U2 Toolkit for .NET V 1.2.1 and start developing some code. You can run almost all samples as it uses sample database ( ‘HS.SALES’ UniVerse Account and ‘demo’ UniData Account)
You can also read U2 Toolkit for .NET V 1.2.1’s MSDN Style Help for information such as Architecture, Account Accessible/Getting Schema, Sample Code etc.
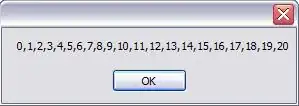
I have tested U2Connection Class’s GetSchema() with UniData’s demo account. It works for me. See screen shot below.

private void button1_Click(object sender, EventArgs e)
{
try
{
U2ConnectionStringBuilder conn_str = new U2ConnectionStringBuilder();
conn_str.UserID = "user";
conn_str.Password = "pass";
conn_str.Server = "localhost";
conn_str.Database = "demo";
conn_str.ServerType = "UNIDATA";
conn_str.Pooling = false;
string s = conn_str.ToString();
U2Connection con = new U2Connection();
con.ConnectionString = s;
con.Open();
this.textBox2.AppendText("Connected......."+Environment.NewLine);
this.textBox2.AppendText("CALLING ....... DataTable dt = con.GetSchema(\"Tables\");"+Environment.NewLine);
DataTable dt = con.GetSchema("Tables");
this.dataGridView1.DataSource = dt.DefaultView;
con.Close();
}
catch (Exception e2)
{
this.textBox2.AppendText(e2.Message);
}
}
It looks like your ‘demo’ account is not normalized. Can you run “sql> select * from SQLTables;” from TCL Command. Do you see the following?
If not, then you can do one of the following:
- Run VSG Tool (Read VSG Manual)
- Run MDM Tool (Read MDM Manual)
Run Command line from TCL Command:
o Convert.sql STUDENT (Read Unidata Manual for convert.sql command)
o Grant privilege
o MIGRATE.SQL
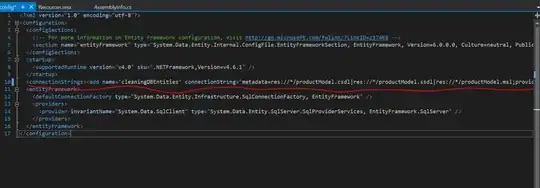