I cannot even see my face without through something reflective. Okay, I can watch myself in a video, but that is done by the optical device called a camera, and it's also something use the technique of reflection.
If I were sick, I possibly would already be dead when doctors need X-rays to diagnose and treat my illness, but all kinds of reflections are unable to see what is private of me. And I can never see myself neither.
The X-ray (or something tomography-like) can watch the inside of me, what I can never do without it.
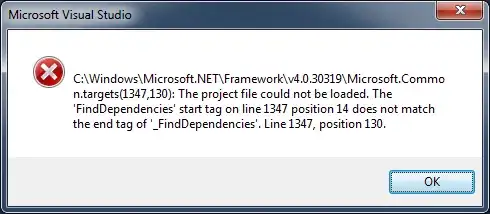
I'd rather say it's more realistic than reflection. But yes, I cannot use my eyes to see the real me directly, every me that I've ever seen, were some kind of reflection. (To think it in depth, eyes also give us the reflection of reality.)
So, reflection is supposed to related to realistic, without a particular view. And you can assume that the consumers code is restricted to BindingFlags.Public, within the rules of object-orientation.
In the real universe, almost nothing is impossible; the only difference between possible and impossible to us, is whether it can done by human, since we are human beings.
It seems dangerous that reflection can do everything in the universe of programs, and now it's required to be fully trusted for the security reason, in a human's logic.