Description
You could do this by combining some recursive logic around a regex
This regex will match open and close brackets nested three layers deep like {a{b{c}}}{{{d}e}f}
\{((?:\{(?:\{.*?\}|.)*?\}|.)*?)\}
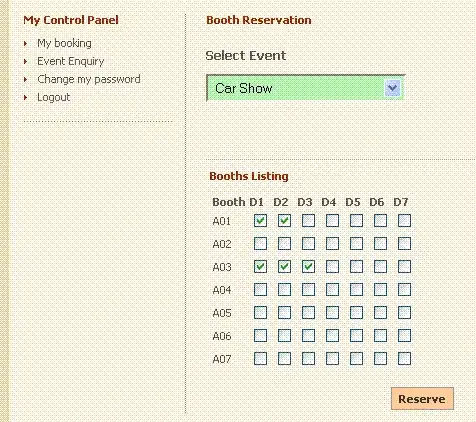
The dotted area is the basic search wherein that search is nested inside itself for as many layers as you need.
In the following example I'm simply running the regex against most of your examples. Combine this regex with a foreach loop that would take each Group 1 and capture all non open brackets from the start of the current string ^[^{]*
, then recursively feed the rest of the string back through the regex above to capture the value inside the next group of brackets, then capture all the non close brackets from the end of the string [^}]*$
.
Sample Text
{a}
{a:b}
{a:{b}}
{a:{b:c}}
{a}{b}
{a}{b}{c}
{a{b{c}}}{{{d}e}f}
C#.NET Code Example:
This C#.Net example is only show how the regex works. See how Group 1 gets the inner text from outer most group of brackets. Each outer bracketed text was broken into it's own array position, and the corresponding outer brackets where removed.
using System;
using System.Text.RegularExpressions;
namespace myapp
{
class Class1
{
static void Main(string[] args)
{
String sourcestring = "sample text above";
Regex re = new Regex(@"\{((?:\{(?:\{.*?\}|.)*?\}|.)*?)\}",RegexOptions.IgnoreCase | RegexOptions.Multiline | RegexOptions.Singleline);
MatchCollection mc = re.Matches(sourcestring);
int mIdx=0;
foreach (Match m in mc)
{
for (int gIdx = 0; gIdx < m.Groups.Count; gIdx++)
{
Console.WriteLine("[{0}][{1}] = {2}", mIdx, re.GetGroupNames()[gIdx], m.Groups[gIdx].Value);
}
mIdx++;
}
}
}
}
$matches Array:
(
[0] => Array
(
[0] => {a}
[1] => {a:b}
[2] => {a:{b}}
[3] => {a:{b:c}}
[4] => {a}
[5] => {b}
[6] => {a}
[7] => {b}
[8] => {c}
[9] => {a{b{c}}}
[10] => {{{d}e}f}
)
[1] => Array
(
[0] => a
[1] => a:b
[2] => a:{b}
[3] => a:{b:c}
[4] => a
[5] => b
[6] => a
[7] => b
[8] => c
[9] => a{b{c}}
[10] => {{d}e}f
)
)
Disclaimer
This expression will only work to the third level of recursion. Outer text will need to be handled separately. The .net regex engine does offer recursion counting and may support N layers deep. As written here this expression may not handle capture g
as expected in {a:{b}g{h}i}
.