Approach 2 (most recent)
If you're absolutely sure you want to use listview("refresh")
, I suggest you use promise()
to resolve whether your append
is completed or not and then apply the refresh
. Like this :
var li = ""; //append to a string
for (var i = 0; i < accounts.length; i++) {
li += '<li data-oid="' + accounts[i]._oid + '"><a><h2>' + accounts[i].nickname + ' - ' + accounts[i].bankname + '</h2><p>Balance: ' + accounts[i].acccy + " " + accounts[i].acbal + '</p></a></li>';
}
//check if append is done by chaining promise() and done()
$('#accountlist').append(li).promise().done(function () {
$(this).listview("refresh"); // refresh here
});
Demo here : http://jsfiddle.net/hungerpain/u9TcE/4/
More info here : Promise Docs
Approach 1
You need not to refresh
listview. listview
elements dont need much css manipulation to make them look like how they look. So they are styled on the fly (ie, when you're append
ing the li
into a ul
with data-role
set to listview
. Look at this array here :
var songs = [
"Sweet Child 'O Mine",
"Summer of '69",
"Smoke in the Water",
"Enter Sandman",
"I thought I've seen everything"
];
Here's the HTML :
<ul data-role="listview" id="songlist" data-theme="c" data-divider-theme="a" data-inset="true">
<li data-role="list-divider" role="heading">Song List</li>
</ul>
Here's how I'm going to inject this :
$.each(songs, function (i, song) {
$("<li/>", {
"data-icon": "star",
"class": "vote",
"html": '<a href="#" id="song' + i + '">' + song + '</a>',
"id": i
}).appendTo("#songlist");
});
THATS IT. You need not do anything more to get this work. The next thing which matters most is placement :
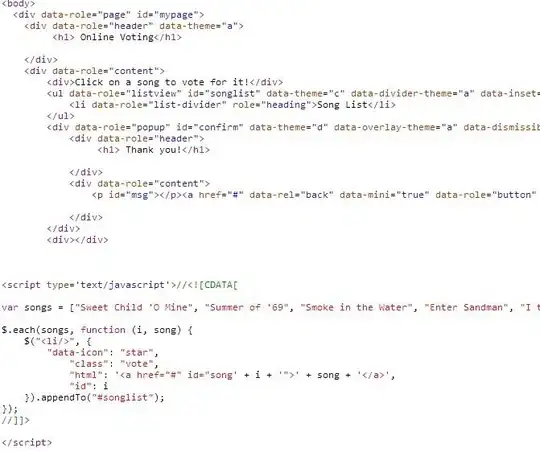
Place your script in body and you're done. The reason this works is because appending is synchronous in <body>
. So you when this is used, you can be sure that the element is already loaded in DOM annd ready to be refreshed, which jQM automatically does.
Here's a demo
The options in jsFiddle - from jsFiddle docs
onLoad: wrap the code so it will run in onLoad window event, place it in <head>
section
onDomReady: wrap the code so it will run in onDomReady window event, place it in <head>
section
no wrap - in <head>
: do not wrap the JavaScript code, place it in <head>
section
no wrap - in <body>
: do not wrap the JavaScript code, place it in <body>
section
For your solution to work, copy the <script>
tag in which you've written this/ the reference of the js
file you've typed this in and paste it as the last element in <body>
.