Description
This regex will capture the attributes type
and detail
regardless of the attribute order, providing they are inside the xt:tag_name
tag.
<xt:tag_name\b(?=\s)(?=(?:(?!\>).)*\s\btype=(["'])((?:(?!\1).)*)\1)(?=(?:(?!\>).)*\s\bdetail=(["'])((?:(?!\3).)*)\3)(?:(?!\>).)*\>
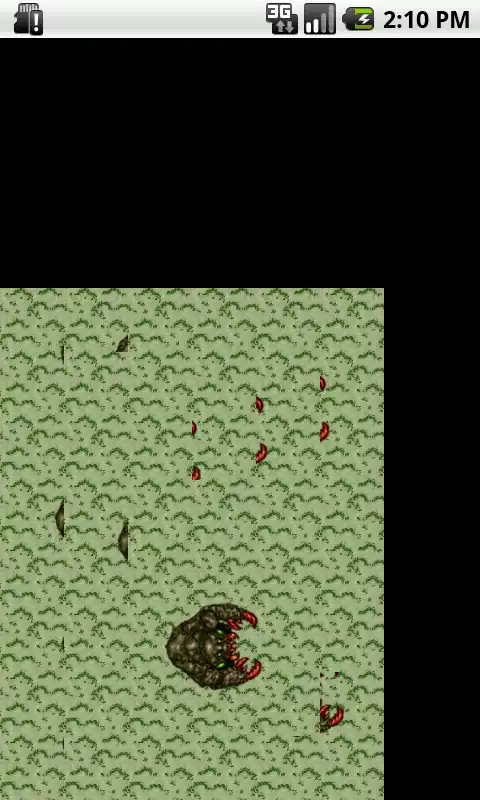
Expanded Description
<xt:tag_name\b
validates the tag name
(?=\s)
ensures there is a space after tag name
(?=
lookahead 1 for the type
. By using a lookahead you can capture the attributes in any order.
(?:(?!\>).)*
move through tag one character at a time and prevent the regex engine from exiting this tag until you reach
\s\btype=
the attribute type
(["'])
capture the open quote, this will be used later to match the proper close tag
((?:(?!\1).)*)
capture all characters inside the quotes, but not including the same type of encapsulating quote
\1
match the close quote
)
close the lookahead for type
(?=(?:(?!\>).)*\s\bdetail=(["'])((?:(?!\3).)*)\3)
does the exact same thing for attribute named detail
as was done for type
(?:(?!\>).)*
match all characters until
\>
the end of the tag
Groups
Group 0 will have the entire tag from the open to close bracket
- will have the open quote around the
type
value, this allows the regex to correctly match the close quote
- will have the value from attribute
type
- will have the open quote around the
detail
value, this allows the regex to correctly match the close quote
- will have the value from attribute
detail
PHP Code Example:
Input string
<xt:tag_name UselessAttribute="some dumb string" type="1" detail="2" /><xt:tag_name detail="Things 'Punk' Loves" MoreUselessAttributes="1231" type="kittens" />
Code
<?php
$sourcestring="your source string";
preg_match_all('/<xt:tag_name\b(?=\s)(?=(?:(?!\>).)*\s\btype=(["\'])((?:(?!\1).)*)\1)(?=(?:(?!\>).)*\s\bdetail=(["\'])((?:(?!\3).)*)\3)(?:(?!\>).)*\>/ims',$sourcestring,$matches);
echo "<pre>".print_r($matches,true);
?>
Matches
$matches Array:
(
[0] => Array
(
[0] => <xt:tag_name UselessAttribute="some dumb string" type="1" detail="2" />
[1] => <xt:tag_name detail="Things 'Punk' Loves" MoreUselessAttributes="1231" type="kittens" />
)
[1] => Array
(
[0] => "
[1] => "
)
[2] => Array
(
[0] => 1
[1] => kittens
)
[3] => Array
(
[0] => "
[1] => "
)
[4] => Array
(
[0] => 2
[1] => Things 'Punk' Loves
)
)