if ((strlen($str) == 1) || (strlen($str) == 0)) {
echo " THIS IS PALINDROME";
}
If strlen($str) <= 1
this is obviously a palindrome.
else {
if (substr($str,0,1) == substr($str,(strlen($str) - 1),1)) {
return Palindrome(substr($str,1,strlen($str) -2));
}
If strlen($str) > 1
and if first and last characters of the string are similar, call the same Palindrome function on the inner string (that is the string without its first and last characters).
else { echo " THIS IS NOT A PALINDROME"; }
}
If first and last characters are not equals, this is not a palindrome.
The principle is to test only the outer characters, and to call the same function again and again on smaller parts of the string, until it has tested every pair of characters that have to be equal if we're dealing with a palindrome.
This is called recursion.
This image illustrates what happens better than my poor english can:
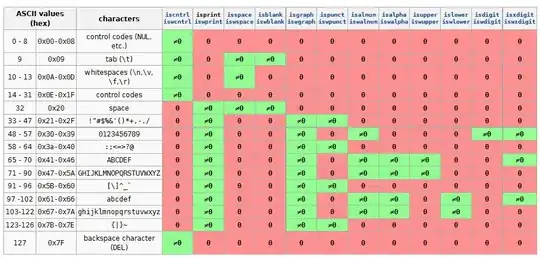
image source