I was looking for the same thing and cobbled something together which may be of assistance to someone else out there.
This is a multi step process, however the result should get you what you need.
Step 1
Create a basic html file containing the following code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Return All Global Variables</title>
</head>
<body>
<script>
var obj = window;
var arr = [];
var arrLength = 0;
do Object.getOwnPropertyNames(obj).forEach(function(name) {
arr.push(name);
});
while(obj = Object.getPrototypeOf(obj));
arrLength = arr.length;
arr.sort();
for (var i = 0; i < arrLength; i++) {
document.write('"' + arr[i] +'"');
if(i < arrLength -1){
document.write(', ');
}
}
</script>
</body>
</html>
Step 2 Run the html file you just created in the browser you are developing in.
You will see a result similar to what is shown below:
"AbortController", "AbortSignal", "AbsoluteOrientationSensor", "AbstractRange", "Accelerometer", "AggregateError", "AnalyserNode", "Animation", "AnimationEffect", "AnimationEvent", "AnimationPlaybackEvent", "AnimationTimeline", "Array", "ArrayBuffer", "Atomics", "Attr", "Audio", "AudioBuffer", "AudioBufferSourceNode", "AudioContext", "AudioData", "AudioDecoder", "AudioDestinationNode", "AudioEncoder", "AudioListener", "AudioNode", "AudioParam", "AudioParamMap", "AudioProcessingEvent", "AudioScheduledSourceNode", "AudioSinkInfo", "AudioWorklet", "AudioWorkletNode", "AuthenticatorAssertionResponse", "AuthenticatorAttestationResponse", "AuthenticatorResponse", "BackgroundFetchManager", "BackgroundFetchRecord", "BackgroundFetchRegistration", "BarProp", "BaseAudioContext", "BatteryManager", "BeforeInstallPromptEvent", "BeforeUnloadEvent", "BigInt", "BigInt64Array", "BigUint64Array", "BiquadFilterNode", "Blob", "BlobEvent", "Bluetooth", "BluetoothCharacteristicProperties", "BluetoothDevice", "BluetoothRemoteGATTCharacteristic", "BluetoothRemoteGATTDescriptor", "BluetoothRemoteGATTServer", "BluetoothRemoteGATTService", "BluetoothUUID", "Boolean", "BroadcastChannel", "BrowserCaptureMediaStreamTrack", "ByteLengthQueuingStrategy", "CDATASection", "CSS", "CSSAnimation", "CSSConditionRule", "CSSContainerRule", "CSSCounterStyleRule", "CSSFontFaceRule", "CSSFontPaletteValuesRule", "CSSGroupingRule", "CSSImageValue", "CSSImportRule", "CSSKeyframeRule", "CSSKeyframesRule", "CSSKeywordValue", "CSSLayerBlockRule", "CSSLayerStatementRule", "CSSMathClamp", "CSSMathInvert", "CSSMathMax", "CSSMathMin", "CSSMathNegate", "CSSMathProduct", "CSSMathSum", "CSSMathValue", "CSSMatrixComponent", "CSSMediaRule", "CSSNamespaceRule", "CSSNumericArray", "CSSNumericValue", "CSSPageRule", "CSSPerspective", "CSSPositionValue", "CSSPropertyRule", "CSSRotate", "CSSRule", "CSSRuleList", "CSSScale", "CSSSkew", "CSSSkewX", "CSSSkewY", "CSSStyleDeclaration", "CSSStyleRule", "CSSStyleSheet", "CSSStyleValue", "CSSSupportsRule", "CSSTransformComponent", "CSSTransformValue", "CSSTransition", "CSSTranslate", "CSSUnitValue", "CSSUnparsedValue", "CSSVariableReferenceValue", "Cache", "CacheStorage", "CanvasCaptureMediaStreamTrack", "CanvasGradient", "CanvasPattern", "CanvasRenderingContext2D", "CaptureController", "ChannelMergerNode", "ChannelSplitterNode", "CharacterData", "Clipboard", "ClipboardEvent", "ClipboardItem", "CloseEvent", "Comment", "CompositionEvent", "CompressionStream", "ConstantSourceNode", "ContentVisibilityAutoStateChangeEvent", "ConvolverNode", "CookieChangeEvent", "CookieStore", "CookieStoreManager", "CountQueuingStrategy", "Credential", "CredentialsContainer", "CropTarget", "Crypto", "CryptoKey", "CustomElementRegistry", "CustomEvent", "CustomStateSet", "DOMError", "DOMException", "DOMImplementation", "DOMMatrix", "DOMMatrixReadOnly", "DOMParser", "DOMPoint", "DOMPointReadOnly", "DOMQuad", "DOMRect", "DOMRectList", "DOMRectReadOnly", "DOMStringList", "DOMStringMap", "DOMTokenList", "DataTransfer", "DataTransferItem", "DataTransferItemList", "DataView", "Date", "DecompressionStream", "DelayNode", "DelegatedInkTrailPresenter", "DeviceMotionEvent", "DeviceMotionEventAcceleration", "DeviceMotionEventRotationRate", "DeviceOrientationEvent", "Document", "DocumentFragment", "DocumentTimeline", "DocumentType", "DragEvent", "DynamicsCompressorNode", "Element", "ElementInternals", "EncodedAudioChunk", "EncodedVideoChunk", "Error", "ErrorEvent", "EvalError", "Event", "EventCounts", "EventSource", "EventTarget", "External", "EyeDropper", "FeaturePolicy", "FederatedCredential", "File", "FileList", "FileReader", "FileSystemDirectoryHandle", "FileSystemFileHandle", "FileSystemHandle", "FileSystemWritableFileStream", "FinalizationRegistry", "Float32Array", "Float64Array", "FocusEvent", "FontData", "FontFace", "FontFaceSetLoadEvent", "FormData", "FormDataEvent", "FragmentDirective", "Function", "GPU", "GPUAdapter", "GPUAdapterInfo", "GPUBindGroup", "GPUBindGroupLayout", "GPUBuffer", "GPUBufferUsage", "GPUCanvasContext", "GPUColorWrite", "GPUCommandBuffer", "GPUCommandEncoder", "GPUCompilationInfo", "GPUCompilationMessage", "GPUComputePassEncoder", "GPUComputePipeline", "GPUDevice", "GPUDeviceLostInfo", "GPUError", "GPUExternalTexture", "GPUInternalError", "GPUMapMode", "GPUOutOfMemoryError", "GPUPipelineError", "GPUPipelineLayout", "GPUQuerySet", "GPUQueue", "GPURenderBundle", "GPURenderBundleEncoder", "GPURenderPassEncoder", "GPURenderPipeline", "GPUSampler", "GPUShaderModule", "GPUShaderStage", "GPUSupportedFeatures", "GPUSupportedLimits", "GPUTexture", "GPUTextureUsage", "GPUTextureView", "GPUUncapturedErrorEvent", "GPUValidationError", "GainNode", "Gamepad", "GamepadButton", "GamepadEvent", "GamepadHapticActuator", "Geolocation", "GeolocationCoordinates", "GeolocationPosition", "GeolocationPositionError", "GravitySensor", "Gyroscope", "HID", "HIDConnectionEvent", "HIDDevice", "HIDInputReportEvent", "HTMLAllCollection", "HTMLAnchorElement", "HTMLAreaElement", "HTMLAudioElement", "HTMLBRElement", "HTMLBaseElement", "HTMLBodyElement", "HTMLButtonElement", "HTMLCanvasElement", "HTMLCollection", "HTMLDListElement", "HTMLDataElement", "HTMLDataListElement", "HTMLDetailsElement", "HTMLDialogElement", "HTMLDirectoryElement", "HTMLDivElement", "HTMLDocument", "HTMLElement", "HTMLEmbedElement", "HTMLFieldSetElement", "HTMLFontElement", "HTMLFormControlsCollection", "HTMLFormElement", "HTMLFrameElement", "HTMLFrameSetElement", "HTMLHRElement", "HTMLHeadElement", "HTMLHeadingElement", "HTMLHtmlElement", "HTMLIFrameElement", "HTMLImageElement", "HTMLInputElement", "HTMLLIElement", "HTMLLabelElement", "HTMLLegendElement", "HTMLLinkElement", "HTMLMapElement", "HTMLMarqueeElement", "HTMLMediaElement", "HTMLMenuElement", "HTMLMetaElement", "HTMLMeterElement", "HTMLModElement", "HTMLOListElement", "HTMLObjectElement", "HTMLOptGroupElement", "HTMLOptionElement", "HTMLOptionsCollection", "HTMLOutputElement", "HTMLParagraphElement", "HTMLParamElement", "HTMLPictureElement", "HTMLPreElement", "HTMLProgressElement", "HTMLQuoteElement", "HTMLScriptElement", "HTMLSelectElement", "HTMLSlotElement", "HTMLSourceElement", "HTMLSpanElement", "HTMLStyleElement", "HTMLTableCaptionElement", "HTMLTableCellElement", "HTMLTableColElement", "HTMLTableElement", "HTMLTableRowElement", "HTMLTableSectionElement", "HTMLTemplateElement", "HTMLTextAreaElement", "HTMLTimeElement", "HTMLTitleElement", "HTMLTrackElement", "HTMLUListElement", "HTMLUnknownElement", "HTMLVideoElement", "HashChangeEvent", "Headers", "Highlight", "HighlightRegistry", "History", "IDBCursor", "IDBCursorWithValue", "IDBDatabase", "IDBFactory", "IDBIndex", "IDBKeyRange", "IDBObjectStore", "IDBOpenDBRequest", "IDBRequest", "IDBTransaction", "IDBVersionChangeEvent", "IIRFilterNode", "IdentityCredential", "IdleDeadline", "IdleDetector", "Image", "ImageBitmap", "ImageBitmapRenderingContext", "ImageCapture", "ImageData", "ImageDecoder", "ImageTrack", "ImageTrackList", "Infinity", "Ink", "InputDeviceCapabilities", "InputDeviceInfo", "InputEvent", "Int16Array", "Int32Array", "Int8Array", "IntersectionObserver", "IntersectionObserverEntry", "Intl", "JSON", "Keyboard", "KeyboardEvent", "KeyboardLayoutMap", "KeyframeEffect", "LargestContentfulPaint", "LaunchParams", "LaunchQueue", "LayoutShift", "LayoutShiftAttribution", "LinearAccelerationSensor", "Location", "Lock", "LockManager", "MIDIAccess", "MIDIConnectionEvent", "MIDIInput", "MIDIInputMap", "MIDIMessageEvent", "MIDIOutput", "MIDIOutputMap", "MIDIPort", "Map", "Math", "MathMLElement", "MediaCapabilities", "MediaDeviceInfo", "MediaDevices", "MediaElementAudioSourceNode", "MediaEncryptedEvent", "MediaError", "MediaKeyMessageEvent", "MediaKeySession", "MediaKeyStatusMap", "MediaKeySystemAccess", "MediaKeys", "MediaList", "MediaMetadata", "MediaQueryList", "MediaQueryListEvent", "MediaRecorder", "MediaSession", "MediaSource", "MediaSourceHandle", "MediaStream", "MediaStreamAudioDestinationNode", "MediaStreamAudioSourceNode", "MediaStreamEvent", "MediaStreamTrack", "MediaStreamTrackEvent", "MediaStreamTrackGenerator", "MediaStreamTrackProcessor", "MessageChannel", "MessageEvent", "MessagePort", "MimeType", "MimeTypeArray", "MouseEvent", "MutationEvent", "MutationObserver", "MutationRecord", "NaN", "NamedNodeMap", "NavigateEvent", "Navigation", "NavigationCurrentEntryChangeEvent", "NavigationDestination", "NavigationHistoryEntry", "NavigationPreloadManager", "NavigationTransition", "Navigator", "NavigatorManagedData", "NavigatorUAData", "NetworkInformation", "Node", "NodeFilter", "NodeIterator", "NodeList", "Notification", "Number", "OTPCredential", "Object", "OfflineAudioCompletionEvent", "OfflineAudioContext", "OffscreenCanvas", "OffscreenCanvasRenderingContext2D", "Option", "OrientationSensor", "OscillatorNode", "OverconstrainedError", "PERSISTENT", "PageTransitionEvent", "PannerNode", "PasswordCredential", "Path2D", "PaymentAddress", "PaymentManager", "PaymentMethodChangeEvent", "PaymentRequest", "PaymentRequestUpdateEvent", "PaymentResponse", "Performance", "PerformanceElementTiming", "PerformanceEntry", "PerformanceEventTiming", "PerformanceLongTaskTiming", "PerformanceMark", "PerformanceMeasure", "PerformanceNavigation", "PerformanceNavigationTiming", "PerformanceObserver", "PerformanceObserverEntryList", "PerformancePaintTiming", "PerformanceResourceTiming", "PerformanceServerTiming", "PerformanceTiming", "PeriodicSyncManager", "PeriodicWave", "PermissionStatus", "Permissions", "PictureInPictureEvent", "PictureInPictureWindow", "Plugin", "PluginArray", "PointerEvent", "PopStateEvent", "Presentation", "PresentationAvailability", "PresentationConnection", "PresentationConnectionAvailableEvent", "PresentationConnectionCloseEvent", "PresentationConnectionList", "PresentationReceiver", "PresentationRequest", "ProcessingInstruction", "Profiler", "ProgressEvent", "Promise", "PromiseRejectionEvent", "Proxy", "PublicKeyCredential", "PushManager", "PushSubscription", "PushSubscriptionOptions", "RTCCertificate", "RTCDTMFSender", "RTCDTMFToneChangeEvent", "RTCDataChannel", "RTCDataChannelEvent", "RTCDtlsTransport", "RTCEncodedAudioFrame", "RTCEncodedVideoFrame", "RTCError", "RTCErrorEvent", "RTCIceCandidate", "RTCIceTransport", "RTCPeerConnection", "RTCPeerConnectionIceErrorEvent", "RTCPeerConnectionIceEvent", "RTCRtpReceiver", "RTCRtpSender", "RTCRtpTransceiver", "RTCSctpTransport", "RTCSessionDescription", "RTCStatsReport", "RTCTrackEvent", "RadioNodeList", "Range", "RangeError", "ReadableByteStreamController", "ReadableStream", "ReadableStreamBYOBReader", "ReadableStreamBYOBRequest", "ReadableStreamDefaultController", "ReadableStreamDefaultReader", "ReferenceError", "Reflect", "RegExp", "RelativeOrientationSensor", "RemotePlayback", "ReportingObserver", "Request", "ResizeObserver", "ResizeObserverEntry", "ResizeObserverSize", "Response", "SVGAElement", "SVGAngle", "SVGAnimateElement", "SVGAnimateMotionElement", "SVGAnimateTransformElement", "SVGAnimatedAngle", "SVGAnimatedBoolean", "SVGAnimatedEnumeration", "SVGAnimatedInteger", "SVGAnimatedLength", "SVGAnimatedLengthList", "SVGAnimatedNumber", "SVGAnimatedNumberList", "SVGAnimatedPreserveAspectRatio", "SVGAnimatedRect", "SVGAnimatedString", "SVGAnimatedTransformList", "SVGAnimationElement", "SVGCircleElement", "SVGClipPathElement", "SVGComponentTransferFunctionElement", "SVGDefsElement", "SVGDescElement", "SVGElement", "SVGEllipseElement", "SVGFEBlendElement", "SVGFEColorMatrixElement", "SVGFEComponentTransferElement", "SVGFECompositeElement", "SVGFEConvolveMatrixElement", "SVGFEDiffuseLightingElement", "SVGFEDisplacementMapElement", "SVGFEDistantLightElement", "SVGFEDropShadowElement", "SVGFEFloodElement", "SVGFEFuncAElement", "SVGFEFuncBElement", "SVGFEFuncGElement", "SVGFEFuncRElement", "SVGFEGaussianBlurElement", "SVGFEImageElement", "SVGFEMergeElement", "SVGFEMergeNodeElement", "SVGFEMorphologyElement", "SVGFEOffsetElement", "SVGFEPointLightElement", "SVGFESpecularLightingElement", "SVGFESpotLightElement", "SVGFETileElement", "SVGFETurbulenceElement", "SVGFilterElement", "SVGForeignObjectElement", "SVGGElement", "SVGGeometryElement", "SVGGradientElement", "SVGGraphicsElement", "SVGImageElement", "SVGLength", "SVGLengthList", "SVGLineElement", "SVGLinearGradientElement", "SVGMPathElement", "SVGMarkerElement", "SVGMaskElement", "SVGMatrix", "SVGMetadataElement", "SVGNumber", "SVGNumberList", "SVGPathElement", "SVGPatternElement", "SVGPoint", "SVGPointList", "SVGPolygonElement", "SVGPolylineElement", "SVGPreserveAspectRatio", "SVGRadialGradientElement", "SVGRect", "SVGRectElement", "SVGSVGElement", "SVGScriptElement", "SVGSetElement", "SVGStopElement", "SVGStringList", "SVGStyleElement", "SVGSwitchElement", "SVGSymbolElement", "SVGTSpanElement", "SVGTextContentElement", "SVGTextElement", "SVGTextPathElement", "SVGTextPositioningElement", "SVGTitleElement", "SVGTransform", "SVGTransformList", "SVGUnitTypes", "SVGUseElement", "SVGViewElement", "Sanitizer", "Scheduler", "Scheduling", "Screen", "ScreenDetailed", "ScreenDetails", "ScreenOrientation", "ScriptProcessorNode", "SecurityPolicyViolationEvent", "Selection", "Sensor", "SensorErrorEvent", "Serial", "SerialPort", "ServiceWorker", "ServiceWorkerContainer", "ServiceWorkerRegistration", "Set", "ShadowRoot", "SharedWorker", "SourceBuffer", "SourceBufferList", "SpeechSynthesisErrorEvent", "SpeechSynthesisEvent", "SpeechSynthesisUtterance", "StaticRange", "StereoPannerNode", "Storage", "StorageEvent", "StorageManager", "String", "StylePropertyMap", "StylePropertyMapReadOnly", "StyleSheet", "StyleSheetList", "SubmitEvent", "SubtleCrypto", "Symbol", "SyncManager", "SyntaxError", "TEMPORARY", "TaskAttributionTiming", "TaskController", "TaskPriorityChangeEvent", "TaskSignal", "Text", "TextDecoder", "TextDecoderStream", "TextEncoder", "TextEncoderStream", "TextEvent", "TextMetrics", "TextTrack", "TextTrackCue", "TextTrackCueList", "TextTrackList", "TimeRanges", "ToggleEvent", "Touch", "TouchEvent", "TouchList", "TrackEvent", "TransformStream", "TransformStreamDefaultController", "TransitionEvent", "TreeWalker", "TrustedHTML", "TrustedScript", "TrustedScriptURL", "TrustedTypePolicy", "TrustedTypePolicyFactory", "TypeError", "UIEvent", "URIError", "URL", "URLPattern", "URLSearchParams", "USB", "USBAlternateInterface", "USBConfiguration", "USBConnectionEvent", "USBDevice", "USBEndpoint", "USBInTransferResult", "USBInterface", "USBIsochronousInTransferPacket", "USBIsochronousInTransferResult", "USBIsochronousOutTransferPacket", "USBIsochronousOutTransferResult", "USBOutTransferResult", "Uint16Array", "Uint32Array", "Uint8Array", "Uint8ClampedArray", "UserActivation", "VTTCue", "ValidityState", "VideoColorSpace", "VideoDecoder", "VideoEncoder", "VideoFrame", "VideoPlaybackQuality", "ViewTransition", "VirtualKeyboard", "VirtualKeyboardGeometryChangeEvent", "VisualViewport", "WakeLock", "WakeLockSentinel", "WaveShaperNode", "WeakMap", "WeakRef", "WeakSet", "WebAssembly", "WebGL2RenderingContext", "WebGLActiveInfo", "WebGLBuffer", "WebGLContextEvent", "WebGLFramebuffer", "WebGLProgram", "WebGLQuery", "WebGLRenderbuffer", "WebGLRenderingContext", "WebGLSampler", "WebGLShader", "WebGLShaderPrecisionFormat", "WebGLSync", "WebGLTexture", "WebGLTransformFeedback", "WebGLUniformLocation", "WebGLVertexArrayObject", "WebKitCSSMatrix", "WebKitMutationObserver", "WebSocket", "WebTransport", "WebTransportBidirectionalStream", "WebTransportDatagramDuplexStream", "WebTransportError", "WheelEvent", "Window", "WindowControlsOverlay", "WindowControlsOverlayGeometryChangeEvent", "Worker", "Worklet", "WritableStream", "WritableStreamDefaultController", "WritableStreamDefaultWriter", "XMLDocument", "XMLHttpRequest", "XMLHttpRequestEventTarget", "XMLHttpRequestUpload", "XMLSerializer", "XPathEvaluator", "XPathExpression", "XPathResult", "XRAnchor", "XRAnchorSet", "XRBoundedReferenceSpace", "XRCPUDepthInformation", "XRCamera", "XRDOMOverlayState", "XRDepthInformation", "XRFrame", "XRHitTestResult", "XRHitTestSource", "XRInputSource", "XRInputSourceArray", "XRInputSourceEvent", "XRInputSourcesChangeEvent", "XRLayer", "XRLightEstimate", "XRLightProbe", "XRPose", "XRRay", "XRReferenceSpace", "XRReferenceSpaceEvent", "XRRenderState", "XRRigidTransform", "XRSession", "XRSessionEvent", "XRSpace", "XRSystem", "XRTransientInputHitTestResult", "XRTransientInputHitTestSource", "XRView", "XRViewerPose", "XRViewport", "XRWebGLBinding", "XRWebGLDepthInformation", "XRWebGLLayer", "XSLTProcessor", "__defineGetter__", "__defineSetter__", "__lookupGetter__", "__lookupSetter__", "__proto__", "addEventListener", "alert", "arr", "arrLength", "atob", "blur", "btoa", "caches", "cancelAnimationFrame", "cancelIdleCallback", "captureEvents", "chrome", "clearInterval", "clearTimeout", "clientInformation", "close", "closed", "confirm", "console", "constructor", "constructor", "constructor", "cookieStore", "createImageBitmap", "credentialless", "crossOriginIsolated", "crypto", "customElements", "decodeURI", "decodeURIComponent", "devicePixelRatio", "dispatchEvent", "document", "encodeURI", "encodeURIComponent", "escape", "eval", "event", "external", "fetch", "find", "focus", "frameElement", "frames", "getComputedStyle", "getScreenDetails", "getSelection", "globalThis", "hasOwnProperty", "history", "i", "indexedDB", "innerHeight", "innerWidth", "isFinite", "isNaN", "isPrototypeOf", "isSecureContext", "launchQueue", "length", "localStorage", "location", "locationbar", "matchMedia", "menubar", "moveBy", "moveTo", "name", "navigation", "navigator", "obj", "offscreenBuffering", "onabort", "onafterprint", "onanimationend", "onanimationiteration", "onanimationstart", "onappinstalled", "onauxclick", "onbeforeinput", "onbeforeinstallprompt", "onbeforematch", "onbeforeprint", "onbeforetoggle", "onbeforeunload", "onbeforexrselect", "onblur", "oncancel", "oncanplay", "oncanplaythrough", "onchange", "onclick", "onclose", "oncontentvisibilityautostatechange", "oncontextlost", "oncontextmenu", "oncontextrestored", "oncuechange", "ondblclick", "ondevicemotion", "ondeviceorientation", "ondeviceorientationabsolute", "ondrag", "ondragend", "ondragenter", "ondragleave", "ondragover", "ondragstart", "ondrop", "ondurationchange", "onemptied", "onended", "onerror", "onfocus", "onformdata", "ongotpointercapture", "onhashchange", "oninput", "oninvalid", "onkeydown", "onkeypress", "onkeyup", "onlanguagechange", "onload", "onloadeddata", "onloadedmetadata", "onloadstart", "onlostpointercapture", "onmessage", "onmessageerror", "onmousedown", "onmouseenter", "onmouseleave", "onmousemove", "onmouseout", "onmouseover", "onmouseup", "onmousewheel", "onoffline", "ononline", "onpagehide", "onpageshow", "onpause", "onplay", "onplaying", "onpointercancel", "onpointerdown", "onpointerenter", "onpointerleave", "onpointermove", "onpointerout", "onpointerover", "onpointerrawupdate", "onpointerup", "onpopstate", "onprogress", "onratechange", "onrejectionhandled", "onreset", "onresize", "onscroll", "onscrollend", "onsearch", "onsecuritypolicyviolation", "onseeked", "onseeking", "onselect", "onselectionchange", "onselectstart", "onslotchange", "onstalled", "onstorage", "onsubmit", "onsuspend", "ontimeupdate", "ontoggle", "ontransitioncancel", "ontransitionend", "ontransitionrun", "ontransitionstart", "onunhandledrejection", "onunload", "onvolumechange", "onwaiting", "onwebkitanimationend", "onwebkitanimationiteration", "onwebkitanimationstart", "onwebkittransitionend", "onwheel", "open", "openDatabase", "opener", "origin", "originAgentCluster", "outerHeight", "outerWidth", "pageXOffset", "pageYOffset", "parent", "parseFloat", "parseInt", "performance", "personalbar", "postMessage", "print", "prompt", "propertyIsEnumerable", "queryLocalFonts", "queueMicrotask", "releaseEvents", "removeEventListener", "reportError", "requestAnimationFrame", "requestIdleCallback", "resizeBy", "resizeTo", "scheduler", "screen", "screenLeft", "screenTop", "screenX", "screenY", "scroll", "scrollBy", "scrollTo", "scrollX", "scrollY", "scrollbars", "self", "sessionStorage", "setInterval", "setTimeout", "showDirectoryPicker", "showOpenFilePicker", "showSaveFilePicker", "speechSynthesis", "status", "statusbar", "stop", "structuredClone", "styleMedia", "toLocaleString", "toString", "toolbar", "top", "trustedTypes", "undefined", "unescape", "valueOf", "visualViewport", "webkitCancelAnimationFrame", "webkitMediaStream", "webkitRTCPeerConnection", "webkitRequestAnimationFrame", "webkitRequestFileSystem", "webkitResolveLocalFileSystemURL", "webkitSpeechGrammar", "webkitSpeechGrammarList", "webkitSpeechRecognition", "webkitSpeechRecognitionError", "webkitSpeechRecognitionEvent", "webkitURL", "window"
The above is an array of all the window propery names.
Step 3
Create a new JavaScript file (myVars.js).
Add the following code:
var windowArray = [insert result from step 2 in here];
var obj = window;
var pageVars = {}; // Page Variables Object
var pageFunctions = []; // Page Functions Array
$(document).ready(function() {
var x = 0;
do Object.getOwnPropertyNames(obj).forEach(function(name) {
if(windowArray.indexOf(name) > -1){
// if the current window object iteration name exists in the windowArray Array, if it does, ignore the result
}else{
if(typeof(window[name]) === "function"){ // check if the object type is a function
if(name.startsWith("$")){ // avoid jquery items
// black hole this result
}else{
pageFunctions.push(name); // add function name to pageFunctions array
}
}else{
if(name.startsWith("jQuery") || name.startsWith("page")){ // filter out any additional non-function page variables which may exist
// black hole this result
}else{
pageVars[name] = window[name]; // add variable to pageVars Object
}
}
}
});
while(obj = Object.getPrototypeOf(obj));
});
Step 4
Add the js file to the bottom of any file you are working on:
<script src="./path/to/myVars.js"></script>
Step 5
- Open the developer tools in the browser you are working in.
- Reload the page you are working on.
- Add pageVars and if required, pageFunctions to the watch list by clicking on the plus button.
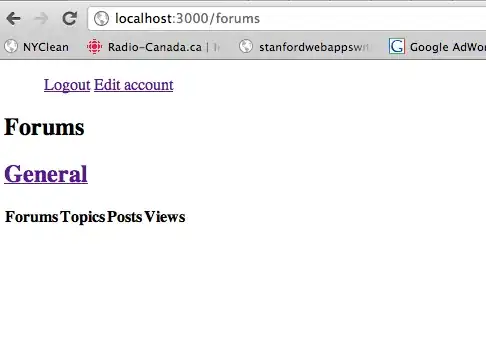
- Press the watch refresh button to make sure all variables are up to date.
You now have a working variable set based on only the variables you have created in your code.
I hope someone finds this useful.
Disclaimer:
This was only tested on chrome and very little debugging and edge case detection was performed.