The ultimate question here isn't which Object Relational Mapper (ORM) to use, but ultimately what such a mapper does. This particular layer is often interlaced into a Data Access Layer (DAL).
You see the function here is essentially a technique to convert between incompatible types. Essentially it creates a Virtual Object Database that can be utilized. The importance of this layer is to link your Domain Logic / Business Layer to your Data Access Layer or Object Relational Mapper. This essentially helps decouple logic that doesn't require access to your data structure- Which makes the decoupling between your User Interface, Domain Logic, and Data Access Layer more coherent.
The Interface has no knowledge of how your Data Layer is speaking to your data structure. Currently I've been mixing the Data Access Layer and Object Relational Mapper due to the similarities but they are quite different.
How is a Data Access Layer different from an Object Relational Mapper
Compared to traditional techniques of exchange between an object-oriented language and a relational database, an Object Relational Mapper often reduces the amount of code that needs to be written. This benefit is apparent- but includes a massive pitfall. They tend to overly abstract what is occuring, which obscures what actually is happening.
Another thing to consider, if you overly rely on the ORM it may produce a poorly designed database.
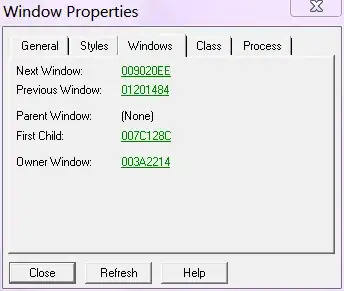
As you can see from the diagram, that is the theory behind an Object Relational Mapper. Where as a Data Access Layer often found within an N-Tier Architecture or Service Oriented Architecture.
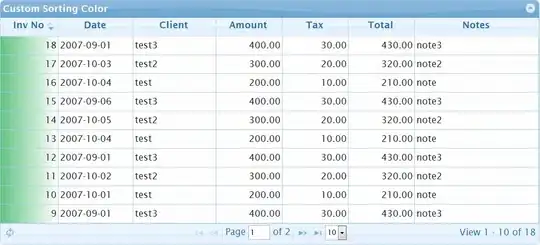
The major difference between the two is:
Object-Relational Mapping tools provide data layers in this fashion,
following the active record model. The ORM/active-record model is
popular with web frameworks.
Where the Data Access Layer is layer which simplifies access to data
stored in a persistent storage of some kind. Applications using a data
access layer can be either database server dependent or independent.
If the data access layer supports multiple database types, the
application becomes able to use whatever databases the DAL can talk
to. In either circumstance, having a data access layer provides a
centralized location for all calls into the database, and thus makes
it easier to port the application to other database systems (assuming
that 100% of the database interaction is done in the DAL for a given
application).
As you can now see the similarities and differences, but what about the data structure? Am I forced within a database? The short answer is no. You have some of these choices:
- Relational Data: You would use a more traditional Database approach.
- NoSQL: Object Relational Mappers* have arose that follow the NoSQL approach.
This will provide greater flexibility based upon the requirements of your project. A wonderful question on Stack Overflow actually has a real-world with a NoSQL approach. (here)
Four highly distinct benefits are:
Productivity: The data access code is usually a significant portion
of a typical application, and the time needed to write that code can
be a significant portion of the overall development schedule. When
using an ORM tool, the amount of code is unlikely to be reduced—in
fact, it might even go up—but the ORM tool generates 100% of the data
access code automatically based on the data model you define, in mere
moments.
Application design: A good ORM tool designed by very experienced
software architects will implement effective design patterns that
almost force you to use good programming practices in an application.
This can help support a clean separation of concerns and independent
development that allows parallel, simultaneous development of
application layers.
Code Reuse: If you create a class library to generate a separate DLL
for the ORM-generated data access code, you can easily reuse the data
objects in a variety of applications. This way, each of the
applications that use the class library need have no data access code
at all.
Application Maintainability: All of the code generated by the ORM is
presumably well-tested, so you usually don’t need to worry about
testing it extensively. Obviously you need to make sure that the code
does what you need, but a widely used ORM is likely to have code
banged on by many developers at all skill levels. Over the long term,
you can refactor the database schema or the model definition without
affecting how the application uses the data objects.
Obviously take those with a grain of salt, because an application is tested doesn't mean it can't be test further or made better.
You can find a large list of Object Relational Mappers:
Those are just a few of the large quantity available, a link with a list. Some are great, some are not. The ultimate goal will be to find which one adheres closely to your desired application goal-
Hopefully that really helps you out.