I need to delete the already set fixed space constraint between B1 and B2
Finding a constraint programmatically
You can either search for B1
in the view constraints, or keep a reference to the NSLayoutConstraint
when you created it. Searching the constraints for B1
is probably less efficient: all the relationship constraints (view to another view) are part of the enclosing superview
. Assuming you have a handle to B1
, you can list all B1
constraints in its superview like so:
// Searching all relationship constraints involving b1
for item in self.view.constraints() {
if let constraint = item as? NSLayoutConstraint {
if let button = constraint.firstItem as? UIButton {
if button == b1 {
println("firstItem found: \(constraint)")
}
}
if let button = constraint.secondItem as? UIButton {
if button == b1 {
println("secondItem found: \(constraint)")
}
}
}
}
Remembering a constraint
By far, the cleaner approach is to keep a reference to the NSLayoutConstraint
you want to later modify or delete. To do this in a Storyboard, create the constraints you need.
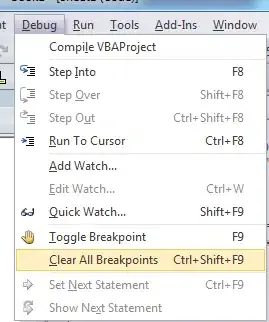
Then control-drag a reference directly into your source file to manipulate them later.
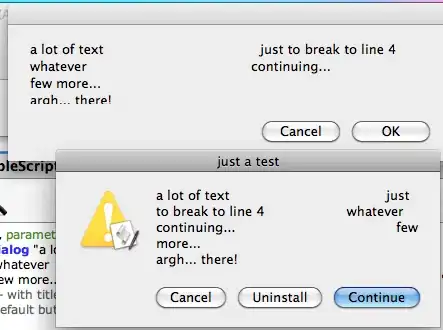