Declare the variable as a instance variable and then initialize Linear Layout
LinearLayout linearLayout;
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment1, container, false);
linearLayout = (LinearLayout) rootView.findViewById(R.id.linearlayout);
return rootView;
}
Then
public void addPlaces() {
Button button = new Button(getActivity());
// needs activity context
// fragment hosted by a activity. use getActivity() to get the context of the hosting activity.
button.setText("button name");
linearlayout.addView(button);
}
Example: Modify the below according to your requirement.
fragment1.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:text="Button" />
<LinearLayout
android:layout_width="fill_parent"
android:id="@+id/linearlayout"
android:layout_height="fill_parent"
android:layout_above="@+id/button1"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:orientation="vertical" >
</LinearLayout>
</RelativeLayout>
Myfragment.java
public class Myfragment extends Fragment {
LinearLayout linearLayout;
View rootView;
@Override
public void onActivityCreated(Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
Button b = (Button) rootView.findViewById(R.id.button1);
b.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
addPlaces();
}
});
linearLayout = (LinearLayout) rootView.findViewById(R.id.linearlayout);
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
rootView = inflater.inflate(R.layout.fragment1, container, false);
return rootView;
}
public void addPlaces() {
Button button = new Button(getActivity()); // needs activity context
button.setText("button name");
linearLayout.addView(button);
}
}
Snap shot of my emulator
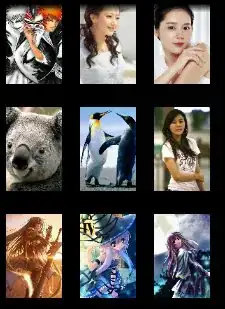
Edit :
activity-main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity" >
<fragment android:name="com.example.fragments.Myfragment"
android:id="@+id/frag"
android:layout_above="@+id/button1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" />
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:text="Button" />
</RelativeLayout>
MainActivity.java
public class MainActivity extends FragmentActivity {
Button b;
Myfragment fragment;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
FragmentManager fragmentManager = getSupportFragmentManager();
FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction();
fragment = new Myfragment();
fragmentTransaction.add(R.id.frag, fragment);
fragmentTransaction.commit();
b = (Button) findViewById(R.id.button1);
b.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
fragment.addPlaces();
}
});
}
}
Myfragment.java
public class Myfragment extends Fragment {
LinearLayout linearLayout;
View rootView;
@Override
public void onActivityCreated(Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
linearLayout = (LinearLayout) rootView.findViewById(R.id.linearlayout);
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
rootView = inflater.inflate(R.layout.fragment1, container, false);
return rootView;
}
public void addPlaces() {
Button button = new Button(getActivity()); // needs activity context
button.setText("button name");
linearLayout.addView(button);
}
}