So, the canvas you are drawing to is in a view that is contained by the RelativeLayout, but you want to draw the dot at the center of the RelativeLayout?
Yes, that is exactly what I am trying to do!
Assuming the canvas view is a direct child of the RealtiveLayout, this should work.
You can get the layout's center by using getWidth() / 2
and getHeight() / 2
on the layout as others mentioned. However, you also have to figure out where the origin of the canvas is. For this you can just use getLeft() and getTop() on the canvas view. Then you just subtract the center x from left, and center y from top to get your final spot.
Example:
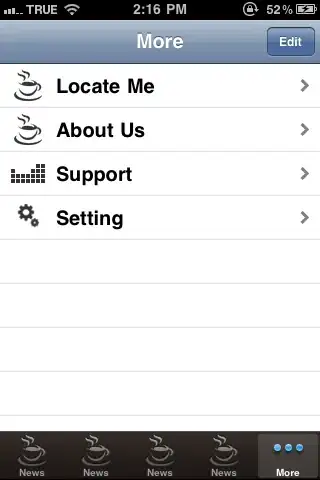
Assume each grid line is 1
. The RelativeLayout
is the large black rectangle, and the Canvas
view is the blue one. The center dot's coordinates are 4,6
. Using left/top, you get 1,4
for the canvas origin(red dot). Subtract, and you get 3,2
, which are the local canvas coordinates for the green dot.