In case someone needed to convert an XML (tabular data) file to XLSX without Microsoft Excel. Here below an NPOI + C# solution....
Sample XML (tabular data):
<rows>
<row>
<col_1>a</col_1>
<col_2>b</col_2>
<col_3>c</col_3>
<col_4>d</col_4>
<col_5>e</col_5>
</row>
<row>
<col_1>f</col_1>
<col_2>h</col_2>
<col_3>h</col_3>
<col_4>i</col_4>
<col_5>j</col_5>
</row>
<row>
<col_1>k</col_1>
<col_2>l</col_2>
<col_3>m</col_3>
<col_4>n</col_4>
<col_5>o</col_5>
</row>
</rows>
C# code:
class clsNpoi
{
public static bool convert_xml_to_xlsx(string xml_path, string row_element_name)
{
try
{
//load xml
XElement elem = XElement.Load(xml_path);
if (elem.XPathSelectElements(row_element_name).Count() == 0)
{
return false; // exit if no row elements are found
}
else
{
//process;
string xpath_text = "//" + row_element_name;
XSSFWorkbook wb = new XSSFWorkbook();
XSSFSheet sh = (XSSFSheet)wb.CreateSheet(Path.GetFileNameWithoutExtension(xml_path));
//get column heads;
var heading_items = elem.XPathSelectElements(xpath_text).First().Elements().Select(e => e.Name.LocalName).Distinct().ToArray();
//get row items;
var row_elems = elem.XPathSelectElements("//" + row_element_name).ToArray();
int row_index = 0, col_index = 0;
foreach (XElement row_elem in row_elems)
{
sh.CreateRow(row_index);
if (row_index == 0)
{
foreach (string heading_item in heading_items)
{
sh.GetRow(row_index).CreateCell(col_index);
sh.GetRow(row_index).GetCell(col_index).SetCellValue(heading_item);
sh.AutoSizeColumn(col_index);
col_index++;
}
row_index++; col_index = 0;
sh.CreateRow(row_index);
}
for (col_index = 0; col_index < heading_items.Count(); col_index++)
{
sh.GetRow(row_index).CreateCell(col_index);
//discard blank / null values -- set "-" as default !important step
var content_value = (string.IsNullOrEmpty(row_elem.Element(heading_items[col_index]).Value) || string.IsNullOrWhiteSpace(row_elem.Element(heading_items[col_index]).Value)) ? "-" : row_elem.Element(heading_items[col_index]).Value;
sh.GetRow(row_index).GetCell(col_index).SetCellValue(content_value);
}
row_index++; col_index = 0;
}
FileStream fs = new FileStream(Path.ChangeExtension(xml_path, ".xlsx"), FileMode.Create, FileAccess.Write);
wb.Write(fs);
fs.Close();
wb.Clear();
return true;
}
}
catch (Exception ex)
{
Debug.Print(ex.ToString());
return false;
}
}
Call as clsNpoi.convert_xml_to_xlsx(<xml_path>, "row");
and the result...
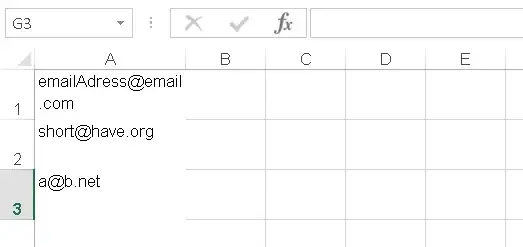
For java usage visit Busy Developers' Guide to HSSF and XSSF Features
For c# usage visit nissl-lab / npoi
Hope this helps someone :)