Something like this? The key point is c=z
which tells scatter
to apply the colormap specified with cmap
to each point according to the scalar value of z
.
import numpy
import matplotlib.pyplot as plt
x=numpy.random.randint(0,100,size=25)
y=numpy.random.randint(0,100,size=25)
z=numpy.random.rand(25)
plt.scatter(x, y, c=z, s=100, cmap=plt.cm.cool, edgecolors='None', alpha=0.75)
plt.colorbar()
plt.show()
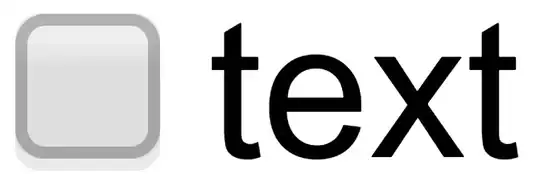
You can also give a list of colors as the c
argument. For example
colors=[(1,0,1,el) for el in z]
plt.scatter(x, y, c=colors, s=100, edgecolors='None')
In this case each point has a RGB tuple associated with it and scatter
uses the color you specify. With the two lines given above you'd have the alpha value of each point vary according to the probability z
. This you can not achieve with just the alpha
keyword of scatter
:
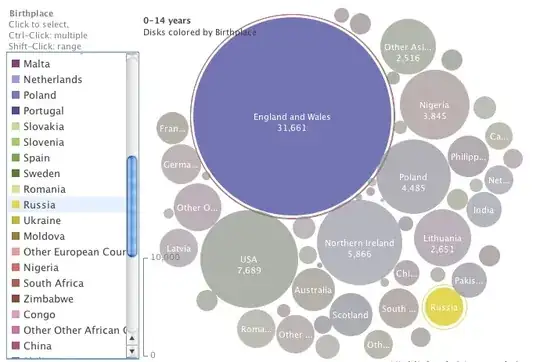