MVC Controller is not ideal for such request handling, but that was the task, so let’s get to it. Let's have an XML that I to accept:
<document>
<id>123456</id>
<content>This is document that I posted...</content>
<author>Michał Białecki</author>
<links>
<link>2345</link>
<link>5678</link>
</links>
</document>
I tried a few solutions with built-in parameter deserialization but none seem to work and finally, I went with deserializing a request in a method body. I created a helper generic class for it:
public static class XmlHelper
{
public static T XmlDeserializeFromString<T>(string objectData)
{
var serializer = new XmlSerializer(typeof(T));
using (var reader = new StringReader(objectData))
{
return (T)serializer.Deserialize(reader);
}
}
}
I decorated my DTO with xml attributes:
[XmlRoot(ElementName = "document", Namespace = "")]
public class DocumentDto
{
[XmlElement(DataType = "string", ElementName = "id")]
public string Id { get; set; }
[XmlElement(DataType = "string", ElementName = "content")]
public string Content { get; set; }
[XmlElement(DataType = "string", ElementName = "author")]
public string Author { get; set; }
[XmlElement(ElementName = "links")]
public LinkDto Links { get; set; }
}
public class LinkDto
{
[XmlElement(ElementName = "link")]
public string[] Link { get; set; }
}
And used all of that in a controller:
public class DocumentsController : Controller
{
// documents/sendDocument
[HttpPost]
public ActionResult SendDocument()
{
try
{
var requestContent = GetRequestContentAsString();
var document = XmlHelper.XmlDeserializeFromString<DocumentDto>(requestContent);
return new HttpStatusCodeResult(HttpStatusCode.OK);
}
catch (System.Exception)
{
// logging
return new HttpStatusCodeResult(HttpStatusCode.InternalServerError);
}
}
private string GetRequestContentAsString()
{
using (var receiveStream = Request.InputStream)
{
using (var readStream = new StreamReader(receiveStream, Encoding.UTF8))
{
return readStream.ReadToEnd();
}
}
}
}
To use it, just send a request using for example Postman. I’m sending POST request to http://yourdomain.com/documents/sendDocument endpoint with xml body mentioned above. One detail worth mentioning is a header. Add Content-Type: text/xml, or request to work.
And it works:
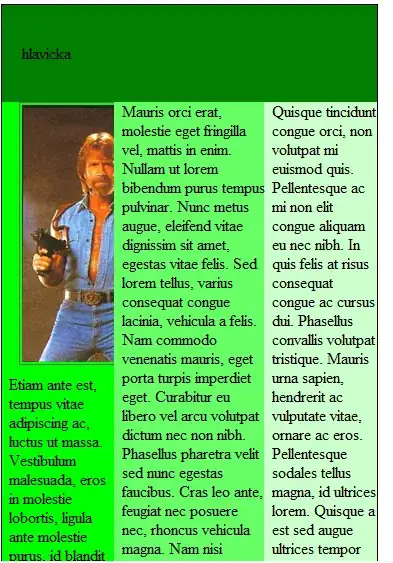
You can see the whole post on my blog: http://www.michalbialecki.com/2018/04/25/accept-xml-request-in-asp-net-mvc-controller/