Add a new hidden UITableView holding your Dynamic cells
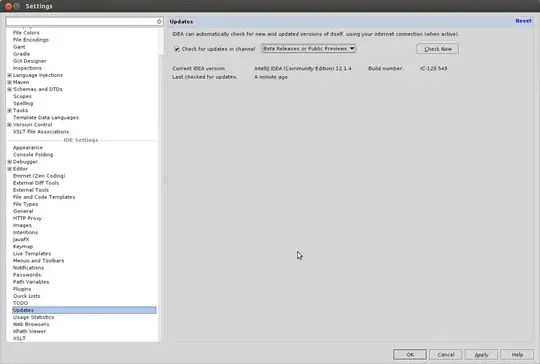
Steps:
1- In your main UITableViewController
append new Section
at the end,
Inside this Section
add only one UITableViewCell
,
Insert a new UITableView
inside the Cell.
let us call the new table tblPrototypes
because
it will be the holder for the Prototype cells.
2- Now set the type of tblPrototypes
to Dynamic Prototypes,
Then add as many prototype UITableViewCell
s as you need.
3- Add an Outlet for tblPrototypes
in the main controller of the Main Static UITableView
,
let us call it tablePrototypes
and of course it is of type UITableView
Coding part:
First make sure to hide tblPrototypes
from the UI,
Since it's the last section in your Main Table, you can do this:
@IBOutlet weak var tblPrototypes: UITableView!
override func numberOfSections(in tableView: UITableView) -> Int {
return super.numberOfSections(in: tableView) - 1
}
The last section will not be presented.
Now when ever you want to display a dynamic cell you do that:
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let needDynamic = true
if !needDynamic {
return super.tableView(tableView, cellForRowAt: indexPath)
}
let cellId = "dynamicCellId1"
let cell = self.tblPrototypes.dequeueReusableCell(withIdentifier: cellId, for: indexPath)
// configure the cell
return cell
}