You need to modify the html for the input field on your webpage, adding a "value" property:
<input value="vin number goes here" />
There are a couple of ways you could achieve this. The best way would be to load the webpage via NSURLConnection, instead of your via your webview, amend the HTML, and then ask the webview to load the amended HTML. Here's an example:
int vinNumber = 1234567890;
NSURLRequest *request = [NSURLRequest requestWithURL:[NSURL URLWithString:@"http://tk14.webng.com/bswbhtml.html"]];
[NSURLConnection sendAsynchronousRequest:request queue:[NSOperationQueue currentQueue] completionHandler:^(NSURLResponse *response, NSData *data, NSError *error) {
if (error == nil && data != nil)
{
NSString *html = [[NSString alloc] initWithData:data encoding:NSUTF8StringEncoding];
if ([html rangeOfString:@"<input type=\"text\" name=\"vinnumber\">"].location != NSNotFound)
{
NSString *amendedInput = [NSString stringWithFormat:@"<input type=\"text\" name=\"vinnumber\" value=\"%i\">", vinNumber];
NSString *amendedHTML = [html stringByReplacingOccurrencesOfString:@"<input type=\"text\" name=\"vinnumber\">" withString:amendedInput];
[_webview loadHTMLString:amendedHTML baseURL:[NSURL URLWithString:@"http://tk14.webng.com/bswbhtml.html"]];
}
}
}];
The resulting page looks like this on my iPhone 4S:
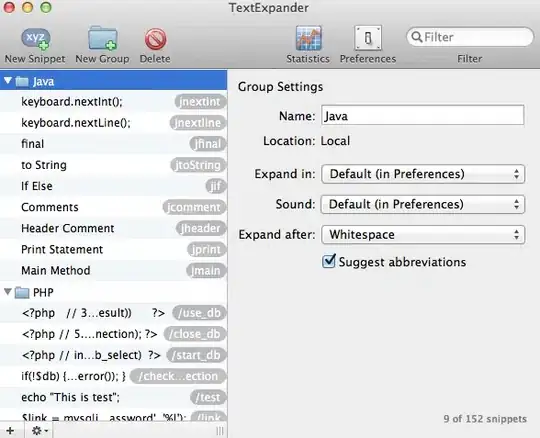