Description
Instead of capturing the individual quoted substrings like in your example, why not do this in one operation, where the offending strings are just replaced while ignoring the other ones.
These expressions will:
- ignore escaped quotes like
"some \"text is quoted\" in here"
- find the desired
~::~
which are either inside or outside the quoted sections which is match is determined by the specific expression.
- assumes the input string already has properly balanced quotes
Note the only difference is with the positive or negative lookahead
Regex: ~::~(?!(?:(?:\\"|[^\\"])*(?:"(?:\\"|[^"])*){2})*$)
This finds the ~::~
which are in side quoted strings
Regex: ~::~(?=(?:(?:\\"|[^\\"])*(?:"(?:\\"|[^"])*){2})*$)
This finds the ~::~
which are out side quoted strings, included here for extra credit but not demonstrated below.
Replace with: empty string
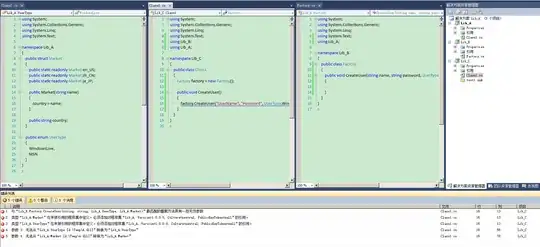
Example
Live Demo In the example, you're interested in the "input.replace()" field which shows the output.
Sample Text
~::~ aaa "bbb" "ccc ~::~ cc\"c ~::~ ccc" "ddd" ~::~ "eee" ~::~
After Replacement
~::~ aaa "bbb" "ccc cc\"c ccc" "ddd" ~::~ "eee" ~::~
Or
If you realy want to just capture the quoted strings while ignoring the escaped quotes then:
"(?:\\"|[^"])*"
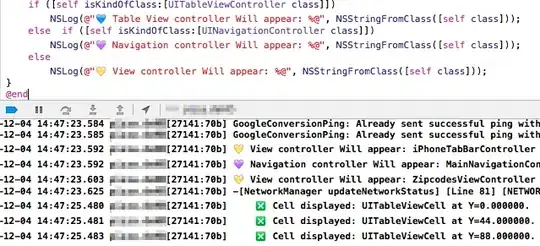
Example
Sample Text
~::~ aaa "bbb" "ccc ~::~ cc\"c ~::~ ccc" "ddd" ~::~ "eee" ~::~
Matches
[0] => "bbb"
[1] => "ccc ~::~ c\"cc ~::~ ccc"
[2] => "ddd"
[3] => "eee"