I added all the explanations into the code:
# Import the things you need
import numpy as np
import matplotlib.pyplot as plt
# Create a matplotlib figure
fig, ax = plt.subplots()
# Create values for the x axis from -pi to pi
x = np.linspace(-np.pi, np.pi, 100)
# Calculate the values on the y axis (just a raised sin function)
y = np.sin(x) + 1
# Plot it
ax.plot(x, y)
# Select the numeric values on the y-axis where you would
# you like your labels to be placed
ax.set_yticks([0, 0.5, 1, 1.5, 2])
# Set your label values (string). Number of label values
# sould be the same as the number of ticks you created in
# the previous step. See @nordev's comment
ax.set_yticklabels(['foo', 'bar', 'baz', 'boo', 'bam'])
Thats it...
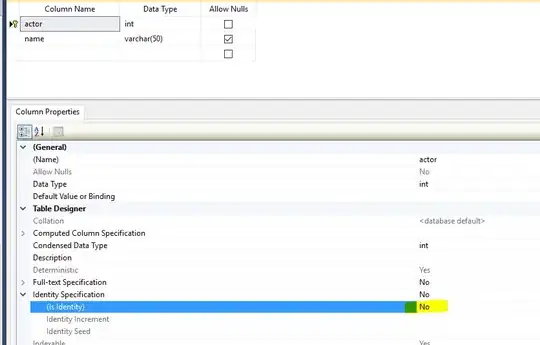
Or if you don't need the subplots just:
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(-np.pi, np.pi, 100)
y = np.sin(x) + 1
plt.plot(x, y)
plt.yticks([0, 0.5, 1, 1.5, 2], ['foo', 'bar', 'baz', 'boo', 'bam'])
This is just a shorter version of doing the same thing if you don't need a figure and subplots.