Regarding C# :
In order for you to run a C#
application from a cmd
, following steps are needed :
- Go to
C:\Windows\Microsoft.NET\Framework\v4.0.30319
location on your File System and copy the path.
- Now right click
Computer
go to Properties.
- Under
System Properties
, select Advanced
Tab, and click on Environment Variables
.
- On
Environment Variables
under User Variables
, select New
.
- For
Variable Name
write CSHARP_HOME
or something else, though I am using the same for further needs to explain this out. For Variable Value
simply Paste
what you copied in Step 1. Click OK.
- Again perform Step 4, if
path
variable does not exist, else you can simply select path
and then click Edit
to perform this next thingy (after putting ;(semi-colon) at the end of the Variable Value
and write %CSHARP_HOME%\
(or use what you used in Step 5) ). This time for Variable Name
write path
, and for Variable Value
use %CSHARP_HOME%\
and click OK.
- Open
cmd
and type csc
and press ENTER, you might be able to see something like this as an output
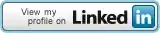
- Now consider I am creating a directory structure for my CSharp Project like this (on File System) at this location
C:\Mine\csharp\command
. Here I created two folders inside command
folder. source and build.
- Now from any
Text Editor
create a small sample program (I am using Notepad++), as below, save it as WinFormExample.cs
under source
folder :
using System;
using System.Drawing;
using System.Windows.Forms;
namespace CSharpGUI {
public class WinFormExample : Form {
private Button button;
public WinFormExample() {
DisplayGUI();
}
private void DisplayGUI() {
this.Name = "WinForm Example";
this.Text = "WinForm Example";
this.Size = new Size(150, 150);
this.StartPosition = FormStartPosition.CenterScreen;
button = new Button();
button.Name = "button";
button.Text = "Click Me!";
button.Size = new Size(this.Width - 50, this.Height - 100);
button.Location = new Point(
(this.Width - button.Width) / 3 ,
(this.Height - button.Height) / 3);
button.Click += new System.EventHandler(this.MyButtonClick);
this.Controls.Add(button);
}
private void MyButtonClick(object source, EventArgs e) {
MessageBox.Show("My First WinForm Application");
}
public static void Main(String[] args) {
Application.Run(new WinFormExample());
}
}
}
- Now type
csc /out:build\WinFormExample.exe source\WinFormExample.cs
(more info is given at the end, for compiler options)and press ENTER to compile as shown below :
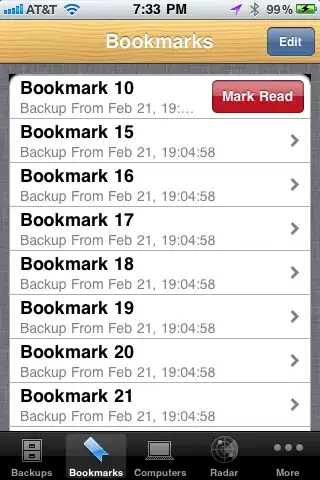
- Now simply run it using
.\build\WinExample
, as shown below :
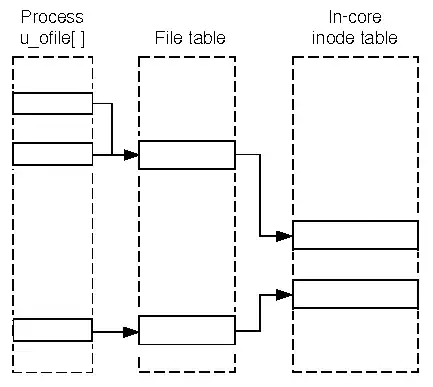
- Now your simple
GUI Application
is up and running :-)
Do let me know, I can explain the same thingy regarding Java
as well, if need be :-)
More info regarding Compiler Options
can be found on C# Compiler Options Listed Alphabetically