On Chromium 89 and Firefox 86 click on body works, except the first click of preload="none"
for Chromium
Of those browsers:
Firefox works perfectly: all clicks on video body toggle play/pause, just like corresponding clicks on controls. Furthermore it shows an intuitive placeholder that makes it clear to the user that clicking will work:

Chromium has a bug where the first click no video body does not work for videos that have preload="none"
. Further clicks work however.
And its placeholder does not make it clear that you can click to play anywhere (which you can't anyways, hopefully they will add a decent placeholder when the bug gets fixed):
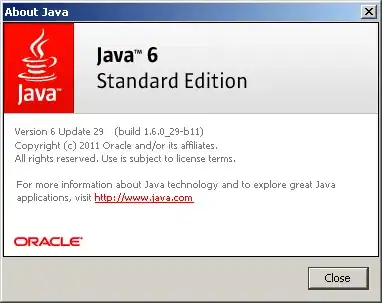
Therefore, the best option is to work around that bug minimally by only doing play on the first click:
<!doctype html>
<html lang=en>
<head>
<meta charset=utf-8>
<title>Min</title>
</head>
<body>
<video id="vid" height="200" controls="controls" preload="none">
<source type="video/webm" src="https://upload.wikimedia.org/wikipedia/commons/transcoded/5/54/Yawning_kitten.ogv/Yawning_kitten.ogv.480p.vp9.webm">
</video>
<script>
let first = true;
document.getElementById('vid').addEventListener('click', (e) => {
if (first) {
e.target.play();
}
first = false;
});
</script>
</body>
</html>
This also does not break Firefox' correct behavior in any way.
TODO: make that workaround perfect by adding an overlay div that clearly indicates that the initial click to play will work, e.g. along the lines of: https://codepen.io/wizaRd_Mockingjay/pen/NVGpep but keeping the controls.
The JavaScript solution shown at: https://stackoverflow.com/a/35563612/895245 of doing onclick="this.play()"
actually breaks the play after the first play + pause from working on those browsers, so I do not recommend it.
The evil part in me wants to think that Chromium behavior is worse because YouTube videos are the only videos that matter to them >:-)