tl;dr
LocalDate // Represent a date, without time-of-day, and without time zone.
.now( ZoneId.systemDefault() ) // Capture the current date as seen in a particular time zone.
.getDayOfWeek() // Returns a `DayOfWeek` enum object.
.getDisplayName( // Generate localized text for the day-of-week’s name.
TextStyle.FULL , // How long or abbreviated should be the day-of-week name.
Locale.getDefault() // The human language and cultural norms to use in localizing.
)
Monday
java.time
Should I use java.util to get system date and time for an android app or should I use the android calender class
Neither. The modern solution is java.time classes.
You asked:
getting the day of the week
Capture the current date as seen in the JVM’s current default time zone.
LocalDate ld = LocalDate.now( ZoneId.systemDefault() ) ;
Extract the day of week.
DayOfWeek dow = ld.getDayOfWeek() ;
Generate localized text for the name of the day of the week.
Locale locale = Locale.getDefault() ;
String output = dow.getDisplayName( TextStyle.FULL , locale ) ;
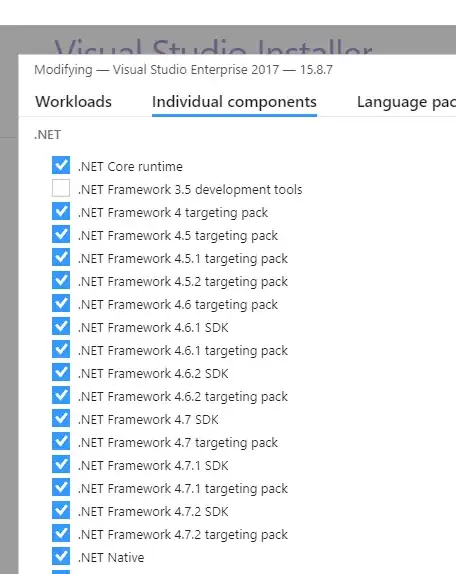
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes. Hibernate 5 & JPA 2.2 support java.time.
Where to obtain the java.time classes?
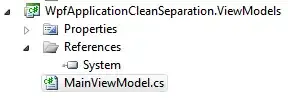