Update
Here is now you use the code below to make a data table:
var newresult = result.SelectMany(cntry => cntry.data.Select(d => new { id = cntry.countryid, name = cntry.countryname, year = d.year, value = d.value }))
.GroupBy(f => f.year)
.Select(g => new { year = g.Key, placeList = g.Select(p => new { p.id, p.value })});
DataTable table = new DataTable();
table.Columns.Add("Year");
foreach(string name in result.Select(x => x.countryid).Distinct())
table.Columns.Add(name);
foreach(var item in newresult)
{
DataRow nr = table.NewRow();
nr["Year"] = item.year;
foreach(var l in item.placeList)
nr[l.id] = l.value;
table.Rows.Add(nr);
}
table.Dump();
And how that looks:
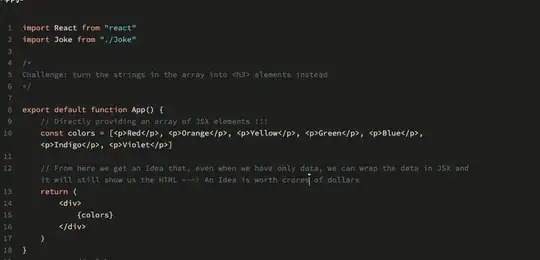
This is what linq can do, you could transform this to a data table easy enough, a list by year of locations and their values.
Flatten the input and then group by. Select what you want. Like this
var newresult = result.SelectMany(cntry => cntry.data.Select(d => new { id = cntry.countryid, name = cntry.countryname, year = d.year, value = d.value }))
.GroupBy(f => f.year)
.Select(g => new { year = g.Key, placeList = g.Select(p => new { p.id, p.value })});
Here is what the dump looks like in LinqPad.
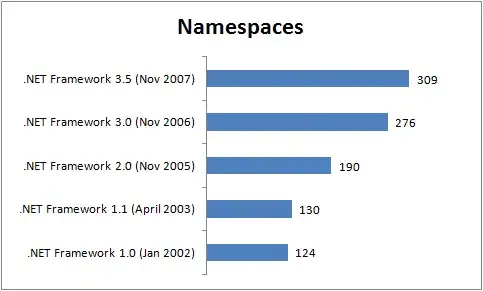
Here is the full test code
void Main()
{
List<cntrydata> result = new List<cntrydata>()
{
new cntrydata() { countryid = "USA", countryname = "United States",
data = new List<Data>() {
new Data() { year = 2000, value = 12 },
new Data() { year = 2001, value = 22 },
new Data() { year = 2004, value = 32 }
}
},
new cntrydata() { countryid = "CAN", countryname = "Canada",
data = new List<Data>() {
new Data() { year = 2001, value = 29 },
new Data() { year = 2003, value = 22 },
new Data() { year = 2004, value = 24 }
}
}
};
var newresult = result.SelectMany(cntry => cntry.data.Select(d => new { id = cntry.countryid, name = cntry.countryname, year = d.year, value = d.value }))
.GroupBy(f => f.year)
.Select(g => new { year = g.Key, placeList = g.Select(p => new { p.id, p.value })});
newresult.Dump();
}
public class cntrydata
{
public string countryid { get; set; }
public string countryname { get; set; }
public IEnumerable<Data> data { get; set; }
}
public class Data
{
public int year { get; set; }
public float value { get; set; }
}